Landing a job at Virtusa, a global information technology (IT) services company, is a coveted goal for many aspiring tech professionals. Known for its innovative solutions and cutting-edge projects, Virtusa seeks individuals with a strong technical foundation, excellent problem-solving skills, and the ability to thrive in a collaborative environment. This comprehensive guide will equip you with the knowledge and strategies needed to navigate the Virtusa interview process and increase your chances of success.
Understanding the Virtusa Interview Process
Virtusa typically employs a multi-stage interview process to evaluate candidates thoroughly. While the exact structure may vary depending on the role and level of experience, you can generally expect the following types of interview rounds:
Technical Round(s): This is the core of the Virtusa interview process. You will be assessed on your technical proficiency in areas relevant to the position you’re applying for. Expect in-depth questions on programming languages, data structures, algorithms, databases, and software engineering principles. You might also face coding challenges, whiteboarding sessions, or technical discussions to demonstrate your problem-solving abilities and coding skills.
HR Round: The HR round focuses on evaluating your soft skills, personality, and cultural fit within the company. Be prepared to answer questions about your teamwork experiences, communication style, leadership qualities, and career aspirations. The HR interview also provides an opportunity for you to ask questions about the company culture, career growth opportunities, and other aspects that are important to you.
Coding/Problem-Solving Assessments: In addition to technical interviews, Virtusa often incorporates coding assessments to evaluate your practical coding skills and problem-solving approach. These assessments may involve solving coding problems on platforms like HackerRank, Codility, or LeetCode, or completing coding challenges during the interview itself.
Virtusa Interview Preparation Key Areas to Focus
To excel in the Virtusa interview process, you need a well-rounded preparation strategy that covers the following key areas:
Technical Proficiency: A strong command of fundamental computer science concepts is crucial. This includes:
- Programming Languages: Be proficient in at least one or two programming languages commonly used at Virtusa, such as Java, C++, Python, or JavaScript. Understand the language’s syntax, data types, control structures, and object-oriented programming (OOP) concepts.
- Data Structures and Algorithms: Master common data structures like arrays, linked lists, stacks, queues, trees, and graphs. Understand their properties, operations, and applications. Familiarize yourself with essential algorithms like searching, sorting, and graph traversal algorithms.
- Databases: If the role requires database knowledge, be prepared to answer questions on relational databases (SQL), database design, and query optimization.
- Software Engineering Principles: Have a good understanding of software development methodologies (Agile, Waterfall), software design patterns, and testing principles.
- Soft Skills and Behavioral Competencies: Virtusa places a strong emphasis on soft skills, as they are essential for effective teamwork, communication, and leadership. Focus on developing and demonstrating the following:
Communication Skills: Articulate your thoughts clearly and concisely. Practice explaining technical concepts in a way that is easy to understand.
- Teamwork and Collaboration: Share examples of how you have effectively collaborated with others to achieve common goals. Highlight your ability to contribute to a team, resolve conflicts, and respect diverse perspectives.
- Problem-Solving and Analytical Skills: Demonstrate your ability to break down complex problems into smaller components, analyze situations, and develop effective solutions.
- Adaptability and Learning Agility: Show that you are open to new challenges, eager to learn new technologies, and can adapt to changing environments
.
Problem-Solving Mindset: Cultivate a structured and analytical approach to problem-solving. Practice the following:
- Understanding the Problem: Take the time to fully understand the problem statement and clarify any ambiguities before jumping into a solution.
- Breaking Down the Problem: Divide complex problems into smaller, more manageable subproblems.
- Developing a Solution: Consider different algorithmic approaches and choose the most efficient one for the given problem.
- Testing and Debugging: Thoroughly test your code and debug any errors.
Virtusa Technical Interview Questions: A Deeper Dive
Questions on Programming Languages (With Answers)
1) Java
1) Explain OOP concepts in Java (e.g., inheritance, polymorphism, encapsulation, abstraction).
Answer:
Object-Oriented Programming (OOP) is a programming paradigm that structures code around objects and data rather than actions and logic. Java is a strongly object-oriented language, and understanding OOP concepts is crucial for any Java developer. Here are some key OOP concepts:
Inheritance: This allows a class (subclass) to inherit properties and methods from another class (superclass). It promotes code reusability and reduces redundancy. For example, you might have a Vehicle class with common properties like color and speed, and subclasses like Car and Motorcycle that inherit these properties and add their own specific attributes.
Polymorphism: This allows objects of different classes to be treated as objects of a common type. It enables flexibility and dynamic method invocation. For instance, both Car and Motorcycle objects can be treated as Vehicle objects. If Vehicle has a method called drive(), both Car and Motorcycle can provide their own specific implementations of this method.
Encapsulation: This involves bundling data (attributes) and methods (functions) that operate on that data within a single unit (class). It helps to hide the internal implementation details of an object and protect its data integrity. Access to the data is controlled through methods (getters and setters).
Abstraction: This allows you to represent complex real-world entities in a simplified manner by hiding unnecessary details. Abstract classes and interfaces are used to define common behaviors without providing a concrete implementation. For example, an interface Drawable might define a method draw(), but the actual implementation of how to draw would be provided by concrete classes like Circle and Rectangle.
2) How does exception handling work in Java?
Answer:
Exception handling in Java provides a mechanism to gracefully handle runtime errors (exceptions) that may occur during program execution. It prevents the program from crashing and allows you to handle errors in a controlled manner. Java uses a try-catch-finally block for exception handling:
try block: The code that might throw an exception is placed within the try block.
catch block: If an exception occurs within the try block, the corresponding catch block that handles that specific exception type is executed. You can have multiple catch blocks to handle different types of exceptions.
finally block: The code within the finally block is executed regardless of whether an exception occurred or not. It’s often used for cleanup tasks like closing files or releasing resources.
Java
try {
// Code that might throw an exception (e.g., file reading, network connection)
} catch (IOException e) {
// Handle IOException
} catch (Exception e) {
// Handle any other exception
} finally {
// Cleanup code (e.g., close file, release resources)
}
Use code with caution.
2) C/C++
1) Explain the difference between pointers and references.
Answer:
Both pointers and references in C/C++ provide ways to work with memory locations and manipulate data indirectly, but they have distinct characteristics:
Pointers:
- Store the memory address of a variable.
- Can be declared without initialization.
- Can be reassigned to point to different variables.
- Can be NULL (pointing to nothing).
- Require explicit dereferencing (* operator) to access the value at the memory address.
References:
- Act as aliases for existing variables.
- Must be initialized when declared.
- Cannot be reassigned to a different variable once initialized.
- Cannot be NULL.
- Do not require explicit dereferencing to access the value.
C++
int x = 10;
int *ptr = &x; // ptr stores the address of x
int &ref = x; // ref is an alias for x
cout << *ptr; // Output: 10 (dereferencing ptr)
cout << ref; // Output: 10
Use code with caution.
2) What are virtual functions and how do they relate to polymorphism?
Answer:
Virtual functions are a key mechanism in C++ to achieve runtime polymorphism. They allow you to define a function in a base class and have derived classes provide their own specialized implementations.
C++
class Shape {
public:
virtual void draw() {
cout << “Drawing a generic shape” << endl;
}
};
class Circle : public Shape {
public:
void draw() override {
cout << “Drawing a circle” << endl;
}
};
class
Rectangle : public Shape {
public:
void draw() override {
cout << “Drawing a rectangle” << endl;
}
};
int main() {
Shape *shapePtr;
Circle circle;
Rectangle rectangle;
shapePtr = &circle;
shapePtr->draw();
// Output: Drawing a circle
shapePtr = &rectangle;
shapePtr->draw(); // Output: Drawing a rectangle
return 0;
}
Use code with caution.
In this example, even though shapePtr is a pointer to the base class Shape, the correct draw() function (from Circle or Rectangle) is called at runtime based on the actual object type. This dynamic binding is enabled by virtual functions.
3) Python
1) Discuss list vs. tuple differences.
Answer:
Lists and tuples are both fundamental data structures in Python used to store collections of items, but they have key differences in terms of mutability and usage:
Lists:
Mutable: You can modify a list after its creation (add, remove, or change elements).
Defined using square brackets [].
Generally used when you need a collection that can change.
Have more built-in methods for modification (e.g., append(), insert(), remove()).
Tuples:
Immutable: Once created, you cannot modify a tuple (add, remove, or change elements).
Defined using parentheses ().
Often used for representing fixed collections of data (e.g., coordinates, database records).
Consume less memory than lists due to their immutability.
Python
my_list = [1, 2, 3]
my_tuple = (1, 2, 3)
my_list[0] = 10 # Valid
# my_tuple[0] = 10 # Invalid – TypeError: ‘tuple’ object does not support item assignment
Use code with caution.
2) How does Python manage memory?
Answer:
Python has automatic memory management, which relieves developers from the burden of manual memory allocation and deallocation. This is achieved through a combination of techniques:
- Reference Counting: Python keeps track of how many references there are to each object. When an object’s reference count drops to zero, it means it’s no longer accessible and its memory is reclaimed.
- Garbage Collection: A garbage collector runs periodically to detect and deallocate objects with cyclical references (objects referencing each other, preventing their reference counts from reaching zero).
- Memory Pooling: Python uses memory pools to efficiently manage memory blocks of different sizes, reducing the overhead of frequent memory allocation and deallocation.
This automatic memory management simplifies development and reduces the risk of memory leaks, but it also means that developers have less direct control over memory usage compared to languages like C/C++.
Data Structures and Algorithms Questions (With Answers)
1) Array-based Questions
1) Find the missing number in a given array of integers from 1 to n, where one number is missing.
Answer:
There are several approaches to solve this problem:
Using Summation:
- Calculate the sum of the numbers from 1 to n using the formula n * (n + 1) / 2.
- Calculate the sum of the elements in the given array.
- Subtract the sum of the array elements from the sum of the first n natural numbers. The result is the missing number.
Using XOR:
- Calculate the XOR of all the numbers from 1 to n.
- Calculate the XOR of all the elements in the array.
- XOR the results from step 1 and step 2. The result is the missing number.
Python
def find_missing_number(arr):
n = len(arr) + 1
total_sum = n * (n + 1) // 2
array_sum = sum(arr)
return total_sum – array_sum
Use code with caution.
2) How would you reverse an array in place?
Answer:
To reverse an array in place (without using extra space), you can use two pointers:
- Initialize a pointer start at the beginning of the array and a pointer end at the end of the array.
- Swap the elements at the start and end pointers.
- Increment the start pointer and decrement the end pointer.
- Repeat steps 2 and 3 until the start pointer is greater than or equal to the end pointer.
Python
def reverse_array(arr):
start = 0
end = len(arr) – 1
while start < end:
arr[start], arr[end] = arr[end], arr[start]
start += 1
end -= 1
Use code with caution.
2) Linked Lists
1) How to detect a cycle in a linked list?
Answer:
The Floyd’s cycle-finding algorithm (also known as the “tortoise and hare” algorithm) is a common method to detect cycles in a linked list:
- Use two pointers, slow and fast. Both start at the head of the list.
- Move the slow pointer one step at a time and the fast pointer two steps at a time.
- If there is a cycle, the fast pointer will eventually meet the slow pointer. If the fast pointer reaches the end of the list (NULL), there is no cycle.
Python
class Node:
def __init__(self, data):
self.data = data
self.next = None
def has_cycle(head):
slow = head
fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
if slow == fast:
return True
return False
Use code with caution.
2) Difference between singly and doubly linked lists.
Answer:
Singly Linked List:
- Each node stores data and a pointer to the next node in the list.
- Traversal is only possible in one direction (forward).
- Simpler implementation.
- Less memory overhead per node.
Doubly Linked List:
- Each node stores data, a pointer to the next node, and a pointer to the previous node.
- Traversal is possible in both directions (forward and backward).
- More complex implementation.
- More memory overhead per node due to the extra pointer.
3) Trees and Graphs
1) Explain tree traversal methods (in-order, pre-order, post-order).
Answer:
Tree traversals are algorithms for visiting all the nodes in a tree in a specific order. Here are the common depth-first traversal methods:
In-order Traversal:
- Visit the left subtree recursively.
- Visit the root node.
- Visit the right subtree recursively.
- Often used for binary search trees to get nodes in ascending order.
Pre-order Traversal:
- Visit the root node.
- Visit the left subtree recursively.
- Visit the right subtree recursively.
- Used for creating a copy of the tree or prefix expression representation.
Post-order Traversal:
- Visit the left subtree recursively.
- Visit the right subtree recursively.
- Visit the root node.
- Used for deleting a tree or postfix expression representation.
2) How would you implement BFS and DFS?
Answer:
BFS (Breadth-First Search):
- Starts at the root (or a designated start node) and explores the graph level by level.
- Uses a queue to keep track of the nodes to be visited.
- Useful for finding the shortest path between two nodes in an unweighted graph.
DFS (Depth-First Search):
- Starts at the root (or a designated start node) and explores as far as possible along each branch before backtracking.
- Uses a stack (or recursion) to keep track of the nodes to be visited.
- Useful for topological sorting, finding connected components, and cycle detection in graphs.
4) Sorting and Searching Algorithms
1) Describe the quicksort algorithm.
Answer:
Quicksort is a divide-and-conquer algorithm. It works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot.
Python
def quicksort(arr):
if len(arr) < 2:
return arr
pivot = arr[0]
less = [i for i in arr[1:] if i <= pivot]
greater = [i for i in arr[1:] if i > pivot]
return quicksort(less)
+ [pivot] + quicksort(greater)
Use code with caution.
2) Explain binary search and its complexity.
Answer:
Binary search is an efficient algorithm for finding a target value within a sorted array. It works by repeatedly dividing the search interval in half.
- Compare the target value to the middle element of the array.
- If the target value matches the middle element, its position is returned.
- If the target value is less than the middle element, the search continues in the left half of the array.
- If the target value is greater than the middle element, the search continues in the right half of the array. 5. This process is repeated until the value is found or the interval is empty.
Binary search has a time complexity of O(log n), which means the number of operations grows logarithmically with the size of the input array, making it very efficient for large datasets.
Python
def binary_search(arr, target):
low = 0
high = len(arr) – 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid – 1
return -1 # Target not found
Use code with caution.
Problem-Solving and Logical Thinking Questions (With Answers)
These questions assess your ability to apply your knowledge to solve real-world problems. They often involve designing algorithms, optimizing solutions, and analyzing their efficiency.
Example 1:
You are given a string. Write a function to check if it is a palindrome.
Answer: A palindrome is a word, phrase, number, or other sequence of characters that reads the same backward as forward. Here’s a Python function to check for palindromes:
Python
def is_palindrome(s):
s = s.lower() # Ignore case
s = ”.join(filter(str.isalnum, s)) # Remove non-alphanumeric characters
return s == s[::-1] # Compare with reversed string
# Example usage
print(is_palindrome(“Racecar”)) # True
print(is_palindrome(“A man, a plan, a canal: Panama”)) # True
Use code with caution.
Example 2:
You are given an array of integers. Write a function to find the maximum sum of a contiguous subarray.
Answer: This is the classic “Maximum Subarray Problem”. Kadane’s algorithm is an efficient solution:
def max_subarray_sum(arr):
max_so_far = 0
max_ending_here = 0
for i in range(len(arr)):
max_ending_here = max_ending_here + arr[i]
if max_ending_here < 0:
max_ending_here = 0
if max_so_far < max_ending_here:
max_so_far = max_ending_here
return max_so_far
“`
Example 3:
Design an algorithm to find the shortest path between two nodes in a weighted graph.
Answer: Dijkstra’s algorithm is a well-known algorithm for finding the shortest paths in a weighted graph with non-negative edge weights. It works by maintaining a set of visited nodes and a distance table that stores the shortest known distance from the source node to each node in the graph.
Virtusa HR Interview Questions: Showcasing Your Soft Skills
The HR interview is your opportunity to demonstrate your personality, communication skills, and alignment with Virtusa’s values. Prepare for questions that explore your experiences, motivations, and career aspirations.
Behavioral Questions (With Answers)
1) Describe a challenging project and how you handled it.
Answer:
Use the STAR method (Situation, Task, Action, Result) to structure your response:
- Situation: Briefly describe the context of the project and the challenge you faced.
- Task: Explain your specific role and responsibilities in the project.
- Action: Describe the actions you took to overcome the challenge. Highlight your problem-solving skills, decision-making process, and any initiative you took.
- Result: Explain the outcome of your efforts. Quantify your achievements whenever possible.
Example:
“In my previous role, we were tasked with developing a new e-commerce platform with a tight deadline. The challenge was that the initial design had some scalability issues. My task was to analyze the design and propose solutions. I conducted performance tests, identified bottlenecks, and suggested using a distributed caching system to improve response times. As a result, we were able to launch the platform on time and handle a significantly higher volume of traffic than initially planned.”
2) Give an example of teamwork in solving a problem.
Answer:
Again, use the STAR method to provide a specific example:
Focus on your contributions to the team, your communication and collaboration skills, and how you effectively worked with others to achieve a shared goal.
Highlight any challenges faced within the team and how they were resolved.
Example:
“During a hackathon, our team was building a mobile app for real-time language translation. We had different ideas on the best approach for the user interface. To resolve this, we organized a brainstorming session where everyone shared their ideas and provided feedback. We then voted on the best approach and worked collaboratively to implement it. This process ensured everyone felt heard and contributed to the final product.”
Questions on Career Motivation and Goals (With Answers)
1) Why are you interested in working at Virtusa?
Answer: Research Virtusa thoroughly before the interview. Understand their mission, values, culture, and the types of projects they undertake. Align your answer with their focus areas and express genuine interest in their work:
Highlight specific aspects of Virtusa that attract you (e.g., their innovative projects, focus on emerging technologies, commitment to employee growth, global presence).
Connect your skills and interests to Virtusa’s needs and explain how you can contribute to their success.
Example:
“I’m particularly interested in Virtusa’s work in digital transformation and cloud computing. I’ve been following your recent projects in the financial services sector, and I’m impressed with your innovative solutions. I believe my skills in [mention your relevant skills] would be a valuable asset to your team, and I’m eager to contribute to Virtusa’s continued growth in these areas.”
2) What are your career goals for the next five years?
Answer: Demonstrate ambition, a clear career path, and a desire for continuous learning. Explain how your goals align with Virtusa’s opportunities for growth and development:
Be specific about your aspirations (e.g., technical expertise, leadership roles, specific domains).
Show that you have a plan for achieving your goals and are willing to invest in your development.
Example:
“In the next five years, I aim to become a technical expert in cloud-native application development. I’m particularly interested in [mention specific cloud technologies]. I see Virtusa as an ideal environment to achieve this goal, as you offer opportunities to work on challenging projects with cutting-edge technologies. I’m also eager to take on leadership responsibilities and mentor junior developers.”
Personal Development and Self-Improvement Questions (With Answers)
1) Describe a situation where you learned from a failure.
Answer: Show self-awareness, a growth mindset, and the ability to learn from your mistakes. Use the STAR method to structure your response:
- Describe the situation and the failure you experienced.
- Explain how you analyzed the situation and identified the reasons for the failure.
- Highlight the specific lessons you learned and how you applied those lessons to improve your performance in the future.
Example:
“In a previous project, I underestimated the complexity of a particular module, which led to delays in the development process. I realized that I had not adequately planned for potential roadblocks and had made some incorrect assumptions. I learned the importance of thorough planning, breaking down tasks into smaller components, and seeking feedback early on. I now use project management tools and techniques to ensure better planning and risk mitigation.”
2) What are your strengths and weaknesses?
Answer: Be honest and self-reflective. Choose a weakness that is not critical for the role and explain how you are working to improve it.
Strengths: Highlight your key skills and qualities that are relevant to the position (e.g., problem-solving, communication, teamwork, adaptability). Provide specific examples to support your claims.
Weaknesses: Choose a weakness that is not a deal-breaker for the role and demonstrate that you are actively working to improve it.
Example:
“One of my strengths is my ability to quickly learn and adapt to new technologies. I’m always eager to explore new tools and frameworks. For example, I recently learned [mention a new technology] in my spare time to expand my skillset. A weakness I’m working on is public speaking. I sometimes get nervous presenting in front of large groups. To improve this, I’ve joined a Toastmasters club and am actively practicing my presentation skills.”
Virtusa Coding/Problem-Solving Assessments: Putting Your Skills to the Test
Virtusa often uses coding assessments to evaluate your practical coding skills and problem-solving abilities. These assessments may be conducted online (using platforms like HackerRank, Codility, or LeetCode) or during the technical interview itself.
Types of Coding/Problem-Solving Questions
Array-based Problems:
- Rotating an array by k elements.
- Finding the missing number in an array.
- Merging two sorted arrays.
- Finding duplicates in an array.
String Manipulation:
- Checking if a string is a palindrome.
- Reversing a string.
- Finding the longest common substring.
- Counting distinct characters in a string.
Dynamic Programming:
- Solving the knapsack problem.
- Finding the longest increasing subsequence.
- Calculating the edit distance between two strings.
Optimization and Complexity Analysis:
- Analyzing the time and space complexity of your solutions.
- Identifying bottlenecks and suggesting improvements for inefficient code.
Tips for Coding Assessments
Practice Regularly: The key to success in coding assessments is consistent practice. Solve problems on platforms like LeetCode, HackerRank, and Codewars. Focus on a variety of problem types and difficulty levels.
Understand Time and Space Complexity: Analyze the time and space complexity of your solutions. Aim for optimal solutions with the lowest possible time and space complexity.
Write Clean and Readable Code: Use meaningful variable names, proper indentation, and comments to make your code easy to understand.
Test Your Code Thoroughly: Before submitting your solution, test it with various test cases, including edge cases and boundary conditions.
Manage Your Time Effectively: During timed assessments, allocate your time wisely. If you get stuck on a problem, move on and come back to it later.
Virtusa Interview Tips for Succeeding : A Holistic Approach
To maximize your chances of success in Virtusa interviews, consider these additional tips:
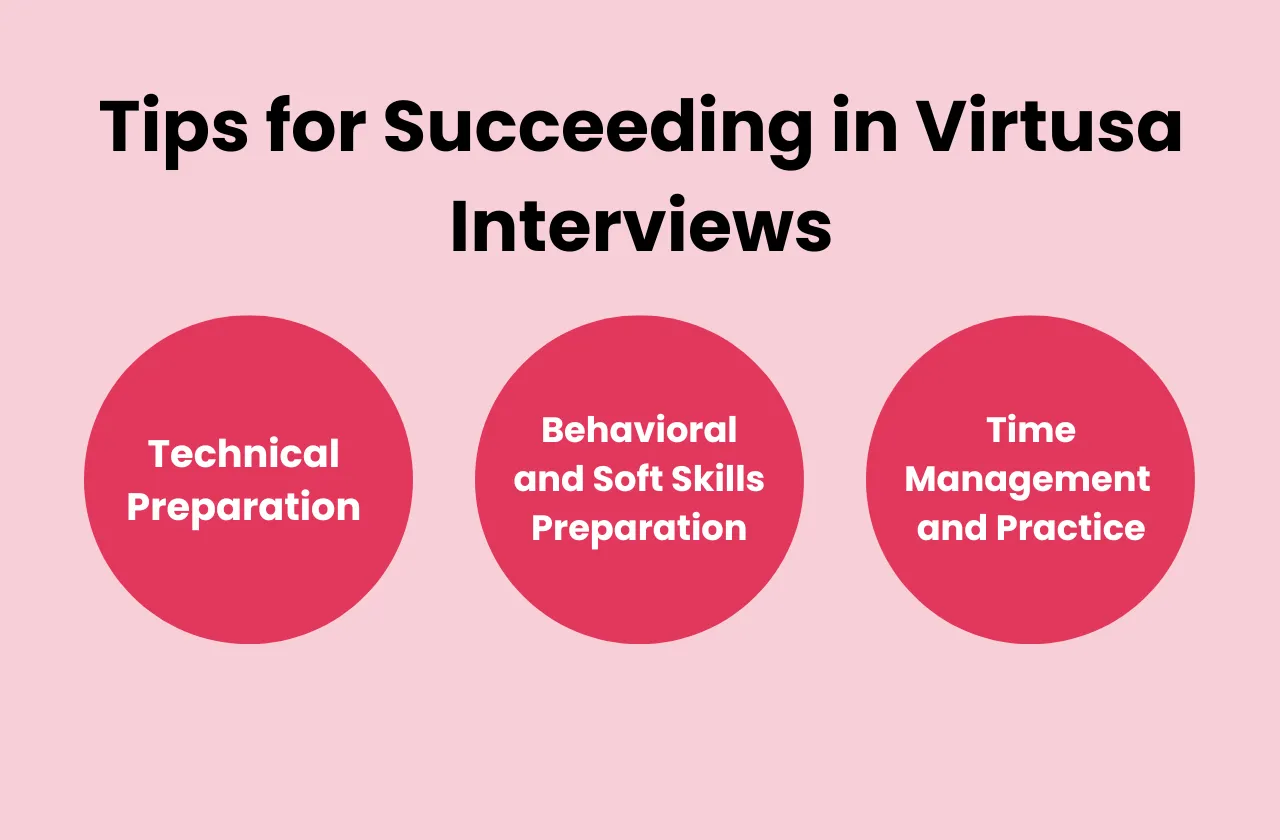
A) Technical Preparation
Master the Fundamentals: A strong foundation in data structures, algorithms, and programming languages is essential.
Familiarize Yourself with Virtusa’s Tech Stack: Research the technologies and tools commonly used at Virtusa. This might include cloud platforms (AWS, Azure, GCP), programming languages (Java, Python, JavaScript), and frameworks (Spring, React, Angular).
Practice Coding on Different Platforms: Get comfortable coding on various platforms, including online coding environments and whiteboards.
Review Past Interview Questions: Look for resources that provide past Virtusa interview questions or questions asked for similar roles in the industry.
B) Behavioral and Soft Skills Preparation
Practice the STAR Method: Use the STAR method to structure your responses to behavioral questions. Prepare examples from your experiences that demonstrate your skills and achievements.
Research Virtusa’s Values: Understand Virtusa’s core values and how they align with your own. Be prepared to discuss how you embody these values in your work.
Display Enthusiasm and a Positive Attitude: Show genuine interest in the company and the role. Express your enthusiasm for contributing to Virtusa’s success.
Prepare Questions to Ask the Interviewer: Asking thoughtful questions demonstrates your curiosity and engagement. Prepare questions about the company culture, projects, career growth opportunities, and other aspects that are important to you.
C) Time Management and Practice
Practice Coding Under Timed Conditions: Simulate the interview environment by practicing coding challenges with time limits. Use online platforms or set a timer for yourself.
Review Your Code and Optimize: After completing a coding challenge, review your code and look for ways to optimize it for time and space complexity.
Get Feedback: If possible, have someone review your code or practice mock interviews with a friend or mentor to get feedback on your performance.
Stay Calm and Confident: Believe in your abilities and approach the interview with a positive attitude. Remember that the interview is a two-way process. It’s an opportunity for you to assess the company as much as it is for them to assess you.
Conclusion
The Virtusa interview process is designed to assess your technical skills, problem-solving abilities, and soft skills. By following this comprehensive guide and dedicating time to thorough preparation, you can increase your chances of success and secure a rewarding career at Virtusa. Remember to focus on building a strong technical foundation, developing your soft skills, and cultivating a problem-solving mindset. Approach the interview with confidence, enthusiasm, and a genuine interest in contributing to Virtusa’s innovative work.
Virtusa Interview FAQs
1) Can you describe your final year project and your role in it?
Interviewers seek insight into your technical skills and teamwork. Clearly explain your project’s objective, the technologies employed, your specific contributions, and the outcomes achieved. Emphasize problem-solving abilities and any innovative approaches you implemented.
2) What is the difference between a list and a set in programming?
A list is an ordered collection allowing duplicate elements, accessible via indices. In contrast, a set is an unordered collection that automatically eliminates duplicates, ensuring all elements are unique. This distinction is crucial when choosing data structures for specific tasks.
3) How would you design a URL shortening service like Bit.ly?
Designing such a service requires creating a system that maps long URLs to shorter ones. Key considerations include generating unique short codes, ensuring scalability to handle numerous requests, implementing efficient database storage, and providing redirection to the original URL upon request.
4) Explain the concept of DNS and its purpose.
The Domain Name System (DNS) translates human-readable domain names into IP addresses (e.g., 192.0.2.1), enabling browsers to load internet resources. It functions as the internet’s phonebook, facilitating user-friendly navigation without memorizing numerical IP addresses.
5) Why do you want to work at Virtusa?
Express your interest by highlighting Virtusa’s innovative projects, commitment to employee development, and its reputation in the tech industry. Align your career goals with the company’s mission and values, demonstrating how you can contribute to and grow within the organization.