Tata Consultancy Services (TCS) is a global leader in IT services, consulting, and business solutions. Its recruitment process is known for its rigour, especially the coding round, which is often one of the most challenging parts of the interview. Coding interviews are designed to test not only a candidate’s technical skills but also their problem-solving abilities, efficiency, and clarity in coding.
The importance of coding skills in the TCS interview process cannot be overstated. Whether you’re applying for a technical role in software development, data analytics, or any other IT-related field, your ability to solve complex coding problems will play a significant role in the decision-making process.
In this article, we’ll provide a comprehensive guide to advanced coding questions and answers that may be asked in TCS interviews. We will cover common coding paradigms, various types of data structures and algorithms, and practical coding tips to help you crack the TCS coding interview.
Understanding TCS Coding Challenges
TCS’s coding challenges are designed to test your understanding of various problem-solving techniques, coding paradigms, and your ability to write efficient and clean code. Let’s start by exploring the types of coding questions typically asked and the most common paradigms you should be familiar with.
Types of Coding Questions Asked in TCS Interviews
In TCS coding interviews, you can expect to face different types of coding challenges. These questions are designed to evaluate your problem-solving skills and your ability to apply algorithms effectively. Here’s a breakdown of the most common types of coding questions:
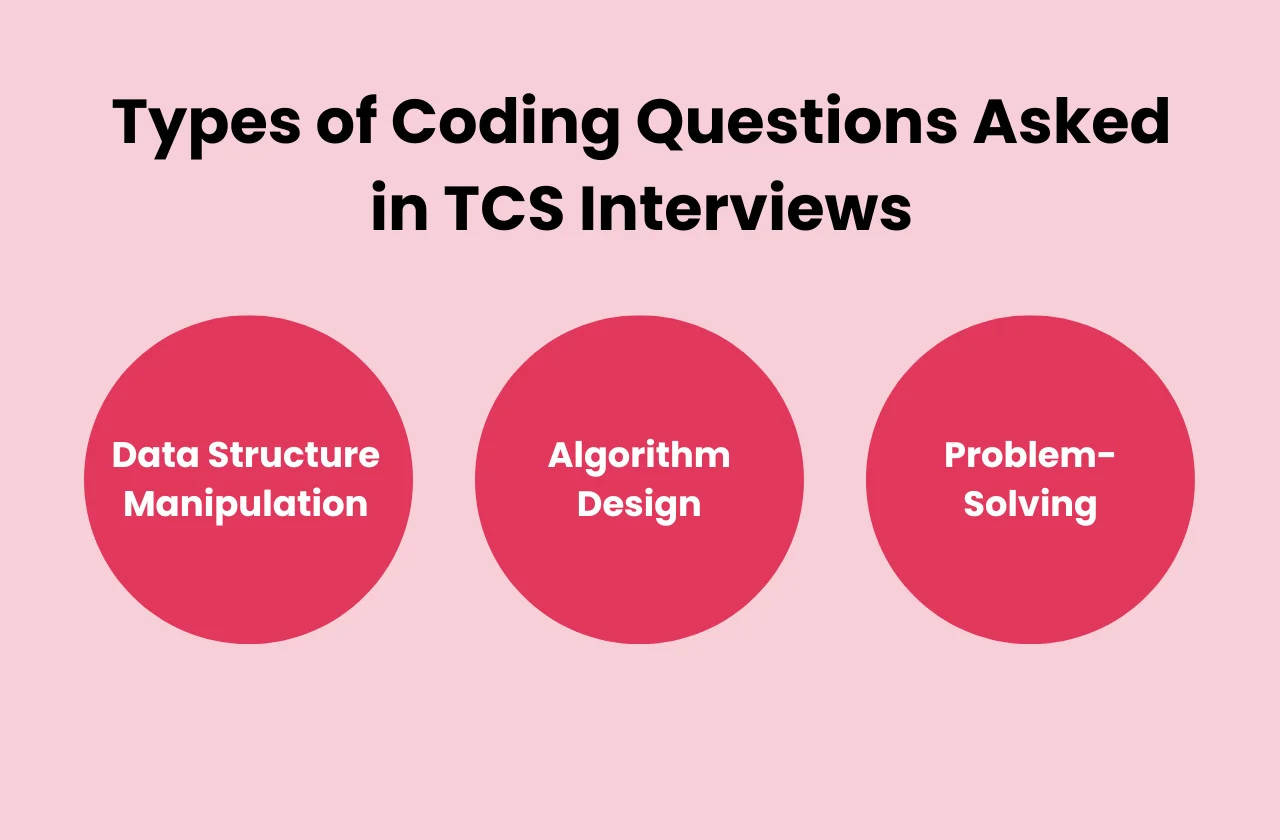
Data Structure Manipulation: These questions will test your understanding of data structures like arrays, linked lists, stacks, queues, trees, graphs, and more. You’ll be asked to perform operations like insertion, deletion, searching, and traversal on these data structures.
Algorithm Design: Algorithm-based questions focus on how well you can design and implement algorithms to solve given problems. These might involve sorting algorithms, searching techniques, optimization problems, and more. Algorithm design also involves choosing the most appropriate algorithm for the problem at hand.
Problem-Solving: These questions present real-world scenarios where you need to solve complex problems using your knowledge of coding and algorithms. These might require a mix of data structures, algorithms, and creativity to arrive at an optimal solution.
Common Coding Paradigms
To successfully tackle coding challenges in TCS interviews, you need to be familiar with several key coding paradigms. Let’s explore some of the most important ones:
Recursion
Recursion is a coding technique where a function calls itself to solve smaller instances of the same problem. Many problems, such as those involving trees and backtracking, can be solved effectively using recursion. However, recursion can be tricky, as it often requires a clear understanding of base cases and recursive cases to avoid infinite loops and ensure optimal performance.
For example, consider the problem of finding the factorial of a number using recursion:
python
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n – 1)
In this example, the function factorial calls itself with a smaller value of n until it reaches the base case where n is 0 or 1.
Dynamic Programming
Dynamic programming is a technique used to solve problems by breaking them down into smaller sub-problems, solving each sub-problem only once, and storing the results for future use. This approach is particularly useful for optimization problems, such as the knapsack problem or the Fibonacci sequence.
For example, here’s a solution to the Fibonacci sequence using dynamic programming:
python
def fibonacci(n):
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i – 1] + dp[i – 2]
return dp[n]
Dynamic programming can significantly reduce the time complexity of algorithms by avoiding redundant calculations.
Greedy Algorithms
A greedy algorithm makes decisions step-by-step, selecting the option that looks the best at each stage, with the hope that this will lead to an optimal solution. Greedy algorithms are used in optimization problems such as the activity selection problem or the fractional knapsack problem.
For example, in the activity selection problem, we aim to select the maximum number of activities that don’t overlap. A greedy approach would involve selecting activities based on their finish times:
python
def activity_selection(activities):
activities.sort(key=lambda x: x[1]) # Sort by finish time
selected = [activities[0]]
last_finish_time = activities[0][1]
for i in range(1, len(activities)):
if activities[i][0] >= last_finish_time:
selected.append(activities[i])
last_finish_time = activities[i][1]
return selected
Greedy algorithms are not always guaranteed to produce the optimal solution, but they often provide efficient and near-optimal solutions to many problems.
Divide and Conquer
Divide and conquer is a strategy where a problem is divided into smaller sub-problems, solved independently, and then combined to get the final solution. This paradigm is commonly used in algorithms like merge sort and quicksort.
For example, here’s an implementation of merge sort using the divide and conquer approach:
python
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left_half = merge_sort(arr[:mid])
right_half = merge_sort(arr[mid:])
return merge(left_half, right_half)
def merge(left, right):
sorted_arr = []
i = j = 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
sorted_arr.append(left[i])
i += 1
else:
sorted_arr.append(right[j])
j += 1
sorted_arr.extend(left[i:])
sorted_arr.extend(right[j:])
return sorted_arr
Divide and conquer algorithms often have efficient time complexities, such as O(n log n) for sorting algorithms like merge sort.
TCS’s Expectations in Terms of Coding Efficiency and Clarity
TCS places a strong emphasis on both coding efficiency and clarity. This means that your code should not only be correct but also optimal and easy to understand. Here are some key points TCS looks for:
Optimal Time Complexity: Your solutions should be designed to run within acceptable time limits. For example, avoid using O(n^2) algorithms when an O(n log n) or O(n) solution exists.
Space Efficiency: Be mindful of the space your code uses. Avoid creating unnecessary data structures or storing redundant information.
Code Readability: Write clean code with clear variable names, proper indentation, and comments where necessary. Readability is key, especially when interviewers ask you to explain your code.
Problem-Solving Approach: Before jumping into writing code, interviewers expect you to first explain your thought process and approach to solving the problem. This gives them insight into how you think and how well you understand the problem.
Now that you have a basic understanding of TCS coding challenges, let’s explore some advanced coding questions and their solutions.
TCS Advanced Coding Questions & Answers
Now, let’s dive into specific coding questions that are commonly asked in TCS interviews. These questions cover a wide range of topics, including data structures, algorithms, and problem-solving techniques.
Data Structures
1) Trees
Question: Write a function to check if a binary tree is balanced.
Solution: A binary tree is balanced if the height of the left and right subtrees of any node differ by no more than one.
python
class Node:
def __init__(self, data):
self.data = data
self.left = None
self.right = None
def height(node):
if node is None:
return 0
return max(height(node.left), height(node.right)) + 1
def is_balanced(root):
if root is None:
return True
left_height = height(root.left)
right_height = height(root.right)
if abs(left_height – right_height) > 1:
return False
return is_balanced(root.left) and is_balanced(root.right)
2) Graphs
Question: Implement Depth First Search (DFS) for a graph.
Solution: DFS is a graph traversal technique that explores as far as possible along a branch before backtracking.
python
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
‘A’: [‘B’, ‘C’],
‘B’: [‘D’, ‘E’],
‘C’: [‘F’],
‘D’: [],
‘E’: [‘F’],
‘F’: []
}
dfs(graph, ‘A’)
3) Heaps
Question: Write a function to implement a max heap.
Solution: A max heap is a binary tree where the value of each node is greater than or equal to the values of its children.
python
import heapq
def heapify(arr):
heapq._heapify_max(arr) # In-place transformation to max heap
def pop_max(arr):
return heapq._heappop_max(arr) # Pops the largest element from the max heap
# Example usage
arr = [3, 5, 9, 6, 8, 20, 10, 12, 18, 9]
heapify(arr)
print(arr) # Outputs the max heap
4) Hash Tables
Question: Write a program to check for anagrams using hash tables.
Solution: Two strings are anagrams if they have the same frequency of characters.
python
def are_anagrams(str1, str2):
if len(str1) != len(str2):
return False
count = {}
for char in str1:
count[char] = count.get(char, 0) + 1
for char in str2:
if char not in count:
return False
count[char] -= 1
if count[char] == 0:
del count[char]
return len(count) == 0
print(are_anagrams(“listen”, “silent”)) # True
Algorithms
1) Sorting Algorithms
Sorting algorithms are a common topic in TCS coding interviews. You might be asked to implement a specific sorting algorithm or compare the time and space complexities of different sorting techniques.
Question: Implement quicksort.
Solution:
python
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
print(quick_sort([3, 6, 8, 10, 1, 2, 1]))
Question: Implement merge sort.
Solution:
python
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left_half = merge_sort(arr[:mid])
right_half = merge_sort(arr[mid:])
return merge(left_half, right_half)
def merge(left, right):
sorted_arr = []
i = j = 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
sorted_arr.append(left[i])
i += 1
else:
sorted_arr.append(right[j])
j += 1
sorted_arr.extend(left[i:])
sorted_arr.extend(right[j:])
return sorted_arr
2) Searching Algorithms
Searching algorithms are another important topic for coding interviews. You should be familiar with both linear search and binary search, as well as their time complexities.
Question: Implement binary search.
Solution:
python
def binary_search(arr, target):
low, high = 0, len(arr) – 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid – 1
return -1
print(binary_search([1, 2, 3, 4, 5, 6, 7], 4)) # Returns 3
3) Dynamic Programming
Dynamic programming problems are common in coding interviews, and you must be comfortable with solving optimization problems using this approach.
Question: Solve the knapsack problem using dynamic programming.
Solution:
python
def knapsack(weights, values, W):
n = len(weights)
dp = [[0 for _ in range(W + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, W + 1):
if weights[i – 1] <= w:
dp[i][w] = max(dp[i – 1][w], dp[i – 1][w – weights[i – 1]] + values[i – 1])
else:
dp[i][w] = dp[i – 1][w]
return dp[n][W]
print(knapsack([1, 3, 4, 5], [1, 4, 5, 7], 7)) # Returns 9
Question: Solve the Fibonacci sequence using dynamic programming.
Solution:
python
def fibonacci(n):
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i – 1] + dp[i – 2]
return dp[n]
print(fibonacci(10)) # Returns 55
4) Greedy Algorithms
Greedy algorithms are often used in optimization problems. You should understand when a greedy approach is appropriate and when it might fail to provide the optimal solution.
Question: Solve the activity selection problem using a greedy algorithm.
Solution:
python
def activity_selection(activities):
activities.sort(key=lambda x: x[1]) # Sort by finish time
selected = [activities[0]]
last_finish_time = activities[0][1]
for i in range(1, len(activities)):
if activities[i][0] >= last_finish_time:
selected.append(activities[i])
last_finish_time = activities[i][1]
return selected
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (8, 9), (5, 9)]
print(activity_selection(activities)) # Optimal selection of activities
Problem-Solving Techniques
In addition to data structures and algorithms, TCS interviews often test your problem-solving techniques. These techniques involve breaking down problems into smaller, manageable parts and systematically solving them.
Divide and Conquer
The divide and conquer technique involves dividing a problem into smaller sub-problems, solving them independently, and then combining the results to solve the original problem. Merge sort is a classic example of this approach.
Backtracking
Backtracking is a problem-solving technique where you build a solution incrementally and abandon solutions as soon as you determine they cannot lead to a valid solution. This technique is commonly used in problems like the N-Queens problem and maze-solving algorithms.
For example, here’s a backtracking solution for solving the N-Queens problem:
python
def solve_n_queens(n):
def is_safe(board, row, col):
for i in range(col):
if board[row][i] == 1:
return False
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0
return False
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0):
for row in board:
print(row)
else:
print(“No solution exists”)
solve_n_queens(4)
Branch and Bound
Branch and bound is an optimization technique where you systematically explore the possible solutions to a problem while pruning branches that are not likely to lead to an optimal solution. This technique is used in problems like the knapsack problem and the travelling salesman problem.
Pattern Recognition
Pattern recognition involves identifying recurring patterns in problems and using these patterns to simplify or solve the problem more efficiently. This technique is useful in problems involving dynamic programming and greedy algorithms.
While practising these questions, it’s crucial to focus on your problem-solving skills and coding efficiency. Let’s move on to some practical tips to help you prepare effectively.
TCS Advanced Coding Interview Preparation Tips
Effective preparation is key to success in TCS coding interviews. This section offers valuable tips to enhance your problem-solving skills, improve your coding efficiency, and boost your confidence.
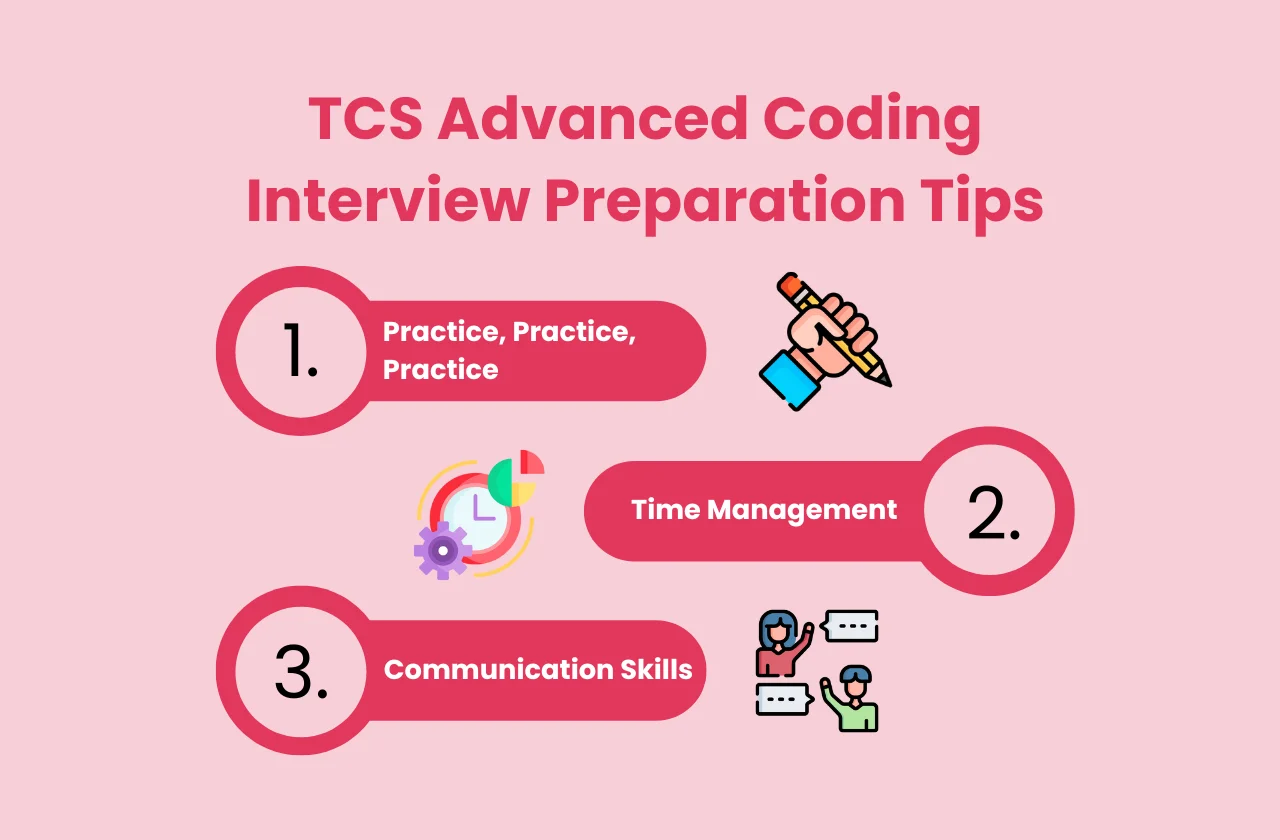
1) Practice, Practice, Practice
The key to success in any coding interview is regular practice. TCS interviews often include challenging coding questions, so it’s essential to practise consistently. Online coding platforms like iScalePro offer a wide range of coding problems that can help you improve your problem-solving skills.
When practising, focus on solving problems in the categories we’ve discussed, including data structures, algorithms, dynamic programming, and greedy algorithms. Make sure you understand the underlying principles behind each solution and how to optimise your code for both time and space complexity.
2) Time Management
Time management is crucial during the coding interview. You will likely face time constraints, so it’s important to practise solving problems within a set time limit. Here are some strategies to help you manage your time effectively:
- Prioritise Easier Problems First: Start with problems you are confident about and solve them quickly. This will give you more time to tackle the more challenging problems later.
- Break Down Complex Problems: If you encounter a difficult problem, break it down into smaller parts and solve each part step by step.
- Avoid Overthinking: Don’t get stuck on one problem for too long. If you’re unsure about a solution, move on to the next problem and return to the difficult one later.
3) Communication Skills
In addition to coding skills, communication is key during the interview process. You should be able to explain your thought process clearly to the interviewer. Here are some tips for improving your communication skills during the interview:
- Explain Your Approach Before Coding: Before you start writing code, explain your approach to solving the problem. This shows the interviewer that you understand the problem and have a plan for solving it.
- Talk Through Your Code: As you write code, explain what you’re doing step by step. This helps the interviewer follow your logic and understand your coding process.
- Ask for Clarification If Needed: If you’re unsure about any part of the problem, don’t hesitate to ask the interviewer for clarification. It’s better to ask questions upfront than to make incorrect assumptions.
Conclusion
TCS coding interviews are designed to test your knowledge of data structures, algorithms, and problem-solving techniques. To succeed, you need to be well-prepared in areas like recursion, dynamic programming, greedy algorithms, and more. Practice regularly, manage your time effectively, and focus on writing clean, efficient code.
By following the tips and solving the problems discussed in this article, you’ll be well on your way to acing the TCS coding interview.