The software testing field is competitive. Landing your dream job requires more than just basic knowledge; it demands a deep understanding of industry-standard tools and best practices. Selenium, a powerful open-source framework for automating web browsers, has become a cornerstone of automated testing for web applications. This comprehensive guide serves as your ultimate companion in preparing for Selenium interviews, equipping you with the knowledge and confidence to impress potential employers. We’ll delve into core concepts, advanced techniques, integration with other tools, and best practices, ensuring you’re well-prepared to tackle any question that comes your way.
Selenium Interview Questions: Core Selenium Concepts
This section focuses on fundamental Selenium concepts that form the bedrock of your automation testing knowledge. Mastering these concepts is crucial for demonstrating a solid foundation to your interviewer.
1) What is Selenium, and why is it popular for automation testing?
Selenium is not just a single tool, but a suite of tools specifically designed for automating web browsers across different platforms. Its open-source nature, combined with support for a wide range of programming languages (Java, Python, C#, Ruby, JavaScript) and operating systems, makes it a highly accessible and versatile choice for testers worldwide.
Selenium’s popularity stems from its ability to automate interactions with web browsers, enabling testers to simulate user actions such as clicking buttons, filling forms, and navigating between pages. This automation capability significantly speeds up the testing process, improves accuracy, and allows for more comprehensive test coverage compared to manual testing.
2) Explain the architecture of Selenium WebDriver.
Selenium WebDriver follows a client-server architecture, facilitating communication between your test scripts and the web browser. Here’s a breakdown of the key components:
Client: This is your test script, written in your chosen programming language, using the Selenium WebDriver API. The client sends commands to the server to perform actions in the browser.
Server: This is the browser-specific driver (e.g., ChromeDriver for Chrome, GeckoDriver for Firefox). It receives commands from the client and executes them within the corresponding browser instance.
The interaction flow is as follows:
- Your test script (client) sends a command, for example, to click a button, using the WebDriver API.
- The browser driver (server) receives this command.
- The driver executes the command within the browser, causing the actual button click to happen.
- The driver then sends the result of the command execution back to the client.
- This architecture allows for seamless interaction with the browser, providing a robust and reliable way to automate web testing.
3) How do you handle pop-ups in Selenium?
Pop-up windows can disrupt the flow of your Selenium tests if not handled correctly. Selenium provides specific methods to interact with different types of pop-ups:
Alert Pop-ups: These are simple JavaScript alert boxes that display a message and require user interaction (e.g., clicking “OK” or “Cancel”). To handle these, use the switchTo().alert() method. This method allows you to access alert-specific methods like accept(), dismiss(), getText(), and sendKeys() to interact with the alert.
Java
Alert alert = driver.switchTo().alert();
String alertText = alert.getText();
alert.accept(); // Clicks “OK”
New Window Pop-ups: When a link or action opens a new browser window, you need to switch Selenium’s focus to this new window. Use the switchTo().window() method along with the window handle to achieve this.
Java
String mainWindowHandle = driver.getWindowHandle();
// Action that opens a new window
for(String windowHandle : driver.getWindowHandles()) {
if(!windowHandle.equals(mainWindowHandle)) {
driver.switchTo().window(windowHandle);
break;
}
}
// Perform
actions in the new window
driver.switchTo().window(mainWindowHandle); // Switch back to the main window
By effectively using these methods, you can ensure your Selenium tests gracefully handle pop-ups and continue executing smoothly.
4) Discuss the differences between Selenium 3 and Selenium 4.
Selenium 4 introduced significant changes and improvements over Selenium 3. Here are some key differences:
W3C Standardization: Selenium 4 adheres to the W3C (World Wide Web Consortium) standard for WebDriver. This standardization eliminates the need for the JSON Wire Protocol used in Selenium 3, resulting in a more stable and reliable interaction between the WebDriver and the browser.
Enhanced Selenium Grid: Selenium Grid, used for distributed testing, has been significantly improved in Selenium 4. It now offers a more efficient and user-friendly architecture, making it easier to set up and manage. Docker support is also simplified.
Relative Locators: Selenium 4 introduces relative locators, allowing you to locate elements based on their position relative to other elements. This makes locating elements easier, especially in dynamic web pages.
Chrome DevTools Protocol Support: Selenium 4 provides native support for the Chrome DevTools Protocol. This gives you more control over the browser, allowing you to simulate network conditions, capture performance metrics, and access advanced browser features.
Deprecation of DesiredCapabilities: While DesiredCapabilities were used in Selenium 3 to configure browser properties, Selenium 4 encourages the use of Options classes for this purpose, providing a more type-safe and structured way to define browser options.
These enhancements in Selenium 4 contribute to a more robust, efficient, and developer-friendly automation framework.
5) How does Selenium handle synchronization issues?
Synchronization issues are a common challenge in Selenium automation. They arise when the web page elements are not loaded or available when your test script tries to interact with them. This can lead to NoSuchElementException or ElementNotInteractableException. Selenium provides various “waits” to address these issues:
Implicit Wait: This tells WebDriver to poll the DOM for a certain amount of time when trying to find an element. If the element is not immediately found, WebDriver will keep trying to locate it within the specified time.
Java
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
Explicit Wait: This allows you to wait for a specific condition to be met before proceeding with the test. You define the condition (e.g., element visibility, clickability) and the maximum wait time.
Java
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“myElement”)));
Fluent Wait: This is a more flexible type of explicit wait. It allows you to configure the polling frequency (how often to check for the condition) and ignore specific exceptions during the wait.
Java
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
WebElement
foo = wait.until(new Function<WebDriver, WebElement>() {
public WebElement apply(WebDriver driver) {
return driver.findElement(By.id(“foo”));
}
});
By effectively utilizing these waits, you can ensure that your Selenium tests are synchronized with the web page, preventing errors and making your tests more reliable.
Once you have a strong grasp of the core concepts, let’s explore some advanced technical questions that you might face.
Selenium Interview Questions: Advanced Technical Questions
This section explores more advanced Selenium concepts, testing your ability to handle complex scenarios and implement robust automation solutions.
6) How do you create a custom framework using Selenium?
Creating a custom Selenium framework provides greater control and flexibility in your automation efforts. Here’s a step-by-step approach:
Choose a Programming Language: Select a language you’re proficient in, such as Java, Python, or C#. This will be the language you use to write your test scripts.
Select a Unit Testing Framework: Choose a unit testing framework that aligns with your chosen language. Popular options include TestNG or JUnit for Java, pytest for Python, and NUnit for C#. These frameworks provide structure for organizing and executing your tests, along with features for assertions and reporting.
Design the Framework Architecture: Plan the structure of your project. This includes:
- Page Object Model (POM): Organize your code by creating page classes representing web pages, encapsulating element locators and actions within those pages.
- Helper Classes: Create utility classes for common functions like reading data from external sources, taking screenshots, or handling waits.
- Configuration Files: Store test data, environment settings, and other configurations in external files (e.g., properties files, XML files) for easy maintenance.
Implement Framework Components:
- Create page classes with locators and methods for interacting with elements on those pages.
- Develop helper classes for common functionalities.
- Set up configuration files to manage test data and environment settings.
Add Reporting and Logging: Integrate reporting libraries (e.g., ExtentReports, Allure) to generate detailed test execution reports. Incorporate logging mechanisms (e.g., Log4j, logging module in Python) to capture important events and debug information during test execution.
Incorporate Version Control: Use a version control system like Git to manage your codebase, track changes, and collaborate effectively with your team.
By following these steps, you can create a custom Selenium framework tailored to your specific needs, promoting code reusability, maintainability, and scalability.
7) Explain the Page Object Model (POM) in Selenium.
The Page Object Model (POM) is a popular design pattern in Selenium that enhances the maintainability and reusability of your test automation code. It promotes a clean separation between page-specific logic and test scripts.
In POM, each web page in your application is represented by a corresponding page class. This class encapsulates the following:
Element Locators: All web elements on that page are defined using locators (e.g., ID, name, XPath) within this class.
Page Actions: Methods representing user interactions with the page elements (e.g., clicking a button, entering text in a field) are defined within the page class.
This approach offers several benefits:
- Maintainability: If the UI of a page changes, you only need to update the locators and methods in the corresponding page class, rather than modifying multiple test scripts.
- Reusability: Page classes can be reused across different test cases, reducing code duplication.
- Readability: POM makes your test scripts more readable and easier to understand, as they focus on the test logic rather than low-level element interactions.
Here’s an example of a simple page class:
Java
public class LoginPage {
private WebDriver driver;
@FindBy(id = “username”)
private WebElement usernameField;
@FindBy(id = “password”)
private WebElement passwordField;
@FindBy(id = “loginBtn”)
private WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void enterUsername(String username)
{
usernameField.sendKeys(username);
}
public void enterPassword(String password) {
passwordField.sendKeys(password);
}
public
void clickLoginButton() {
loginButton.click();
}
}
8) How do you perform data-driven testing with Selenium?
Data-driven testing involves separating test data from your test scripts, allowing you to run the same test with different data sets. This approach increases test coverage and makes your tests more flexible.
Here’s how you can implement data-driven testing in Selenium:
Store Test Data: Store your test data in external sources such as:
- Excel files: Use Apache POI library to read data from Excel sheets.
- CSV files: Use libraries like OpenCSV to read data from CSV files.
- Databases: Use JDBC to connect to a database and retrieve data.
- Read Test Data: In your Selenium test script, use the appropriate library to read the test data from your chosen source.
Parameterize Your Test: Use the data read from the external source to parameterize your test script. This means using the data to populate input fields, make assertions, or control the flow of your test.
Here’s an example using TestNG and an Excel file:
Java
@DataProvider(name = “loginData”)
public Object[][] getLoginData() throws IOException {
// Code to read data from Excel file using Apache POI
// …
return data; // data is a 2D array containing the test data
}
@Test(dataProvider = “loginData”)
public void testLogin(String username, String password, String expectedResult) {
// … Selenium test logic …
loginPage.enterUsername(username);
loginPage.enterPassword(password);
loginPage.clickLoginButton();
// … Assert the result based on expectedResult …
}
9) Describe how to use DesiredCapabilities in Selenium.
While DesiredCapabilities were more prominent in Selenium 3, they are still relevant in certain situations, even though Selenium 4 encourages the use of Options classes. DesiredCapabilities allow you to define browser properties and configure the WebDriver’s behavior. These are key-value pairs that you can use to:
- Specify the browser name and version (e.g., Chrome, Firefox, Edge).
- Set the operating system for the browser environment.
- Enable or disable browser features (e.g., JavaScript, cookies).
- Set proxy settings.
Here’s an example of setting DesiredCapabilities for Chrome:
Java
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setBrowserName(“chrome”);
ChromeOptions options = new ChromeOptions();
options.addArguments(“–headless”);
// Run Chrome in headless mode
capabilities.setCapability(ChromeOptions.CAPABILITY, options);
WebDriver driver = new ChromeDriver(capabilities);
In Selenium 4, you would typically use the Options class for the specific browser (e.g., ChromeOptions, FirefoxOptions) to configure these settings.
10) What is Fluent Wait, and how does it differ from Explicit Wait?
Both Fluent Wait and Explicit Wait are types of waits in Selenium that allow you to wait for a specific condition to be met before proceeding with your test. However, Fluent Wait offers more flexibility and customization compared to Explicit Wait.
Here’s a breakdown of their key differences:
Polling Frequency: Fluent Wait allows you to specify the frequency at which WebDriver checks for the condition. This means you can control how often WebDriver polls the DOM. Explicit Wait, by default, polls every 500 milliseconds.
Ignoring Exceptions: Fluent Wait allows you to ignore specific exceptions while waiting for the condition.
This is useful when you expect certain exceptions to be thrown during the wait and want to continue waiting. Explicit Wait does not have this capability.
Fluent Wait is particularly useful in situations where:
- The element you’re waiting for takes a variable amount of time to load.
- You want to handle specific exceptions during the wait.
- You need more fine-grained control over the polling frequency.
Here’s an example of using Fluent Wait:
Java
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofMillis(500))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(driver -> driver.findElement(By.id(“myElement”)));
Besides technical skills, understanding how Selenium integrates with other tools and technologies is crucial. Let’s discuss some questions related to Selenium’s integration capabilities.
Selenium Interview Questions: Integration and Toolchain
This section focuses on Selenium’s integration with other tools and technologies, highlighting your ability to work with industry-standard development and testing ecosystems.
11) How do you integrate Selenium with CI/CD tools like Jenkins?
Integrating Selenium with Continuous Integration/Continuous Delivery (CI/CD) tools like Jenkins is essential for automating your testing process and enabling faster feedback cycles. Here’s how you can achieve this:
Install Necessary Plugins: In Jenkins, install plugins like the “Maven Integration plugin” or “Git plugin” to enable interaction with your source code repository and build tools.
Configure a Jenkins Job: Create a new Jenkins job and configure it to:
- Source Code Management: Connect to your source code repository (e.g., Git) to check out the latest code.
- Build: Use a build tool like Maven or Gradle to compile your Selenium project and generate the test executables.
- Execute Tests: Configure the job to execute your Selenium tests using the appropriate commands (e.g., mvn test).
- Post-Build Actions: Archive test results, generate reports (using plugins like “HTML Publisher plugin”), and send notifications (e.g., email) about the test execution status.
- Trigger the Job: Configure the job to trigger automatically based on events like code commits, pull requests, or on a scheduled basis.
This integration allows you to run your Selenium tests automatically as part of your CI/CD pipeline, ensuring that code changes are continuously tested and that any issues are identified early in the development cycle.
12) What is the role of Maven in Selenium projects?
Maven is a powerful build automation tool that plays a crucial role in managing dependencies and building Selenium projects. Here’s how Maven benefits your Selenium projects:
Dependency Management: Maven simplifies the process of managing external libraries (dependencies) that your Selenium project relies on. You define your project’s dependencies in the pom.xml file, and Maven automatically downloads and includes them in your project. This eliminates the need for manual downloads and ensures consistent dependency management across your team.
Build Automation: Maven provides a standardized way to build your Selenium project. It handles the compilation, testing, and packaging of your code, ensuring a consistent and repeatable build process.
XML
<project>
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.12.0</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.8.0</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.1.2</version>
<configuration>
<suiteXmlFiles>
<suiteXmlFile>testng.xml</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
</project>
Project Structure: Maven enforces a standard directory structure for your project, promoting organization and consistency.
Plugins: Maven provides a wide range of plugins for various tasks, including running tests, generating reports, and deploying your application.
By using Maven in your Selenium projects, you streamline your build process, improve dependency management, and ensure a more structured and maintainable project.
13) How do you connect Selenium with a database for testing?
Connecting Selenium with a database allows you to perform database testing as part of your automation efforts. This is useful for verifying data integrity, validating data updates, or populating test data. Here’s how you can connect Selenium with a database:
Include JDBC Driver: Add the JDBC driver for your database (e.g., MySQL Connector/J for MySQL) as a dependency in your project.
Establish a Connection: Use the DriverManager.getConnection() method to establish a connection to your database. You’ll need to provide the database URL, username, and password.
Java
Connection connection = DriverManager.getConnection(“jdbc:mysql://localhost:3306/mydatabase”, “username”, “password”);
Execute Queries: Create a Statement object and use it to execute SQL queries against the database.
Java
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(“SELECT * FROM
users”);
Process Results: Retrieve and process the data from the ResultSet object.
Close the Connection: Close the database connection when you’re finished.
Java
resultSet.close();
statement.close();
connection.close();
By integrating Selenium with database testing, you can ensure that your application’s data layer is functioning correctly and that data integrity is maintained.
14) Explain the integration of Selenium with Docker.
Docker is a containerization platform that allows you to package your application and its dependencies into isolated containers. This integration offers several benefits for Selenium testing:
Consistent Environment: Docker ensures that your Selenium tests run in a consistent environment across different machines, regardless of the underlying operating system or configurations. This eliminates environment-related inconsistencies that can lead to flaky tests.
Simplified Setup: Docker simplifies the setup of your testing environment. You can define all the necessary software (browser, WebDriver, dependencies) in a Dockerfile, and Docker will build an image containing everything your tests need.
Parallel Execution: Docker containers can be easily spun up and down, making it ideal for parallel test execution. You can run multiple containers simultaneously, each with a different browser or configuration, significantly reducing test execution time.
Scalability: Docker’s containerization approach makes it easy to scale your testing infrastructure. You can easily deploy and manage multiple containers across different machines or cloud environments.
Here’s a basic example of a Dockerfile for Selenium with Chrome:
Dockerfile
FROM selenium/standalone-chrome:latest
# Add your test scripts to the container
COPY . /tests
# Set the working directory
WORKDIR /tests
# Run your tests
CMD [“mvn”, “test”]
15) Discuss Selenium’s compatibility with cloud-based platforms like Sauce Labs or BrowserStack.
Selenium’s compatibility with cloud-based testing platforms like Sauce Labs and BrowserStack provides significant advantages for your testing efforts:
Wide Range of Environments: These platforms offer a vast collection of browsers, operating systems, and devices, allowing you to test your web application across different configurations without the need to maintain your own infrastructure.
Scalability and Flexibility: Cloud-based platforms provide on-demand scalability, allowing you to run tests in parallel across multiple environments and scale your testing efforts as needed.
Reduced Maintenance: You don’t need to worry about setting up, maintaining, or updating your own testing infrastructure. The cloud provider handles all of this.
Enhanced Collaboration: Cloud platforms often provide features for collaboration, allowing teams to share test results, track progress, and work together more effectively.
To use Selenium with these platforms, you typically need to:
- Configure your tests: Set the WebDriver capabilities to point to the cloud provider’s Selenium Grid.
- Provide credentials: Use your cloud platform credentials to authenticate your tests.
- Execute tests: Run your Selenium tests, and they will be executed on the cloud platform’s infrastructure.
This integration with cloud-based platforms significantly expands your testing capabilities and allows you to achieve broader test coverage.
Debugging and troubleshooting are essential skills for any automation engineer. Let’s explore some common debugging and troubleshooting questions.
Selenium Interview Questions: Debugging and Troubleshooting
This section focuses on your problem-solving skills and your ability to handle common challenges in Selenium automation.
16) How do you debug flaky tests in Selenium?
Flaky tests are tests that pass sometimes and fail other times without any code changes. They are a major source of frustration and can erode confidence in your test suite. Here’s a systematic approach to debugging flaky tests:
Isolate the Test: Identify the specific test that is flaky. Run it multiple times to confirm its inconsistent behavior.
Analyze Test Logs: Examine the test logs and any error messages to understand the failure points. Look for patterns or clues that might indicate the cause of the flakiness.
Check for Timing Issues: Timing issues are a common cause of flaky tests. Use explicit waits to ensure that elements are loaded before interacting with them. Avoid using Thread.sleep, as it can introduce unpredictable delays.
Inspect for Race Conditions: Race conditions occur when multiple threads or processes access shared resources concurrently, leading to unpredictable behavior. Analyze your test for any potential race conditions, especially if you’re performing parallel testing.
Review Test Dependencies: If your test depends on other tests or external factors, ensure that these dependencies are stable and reliable.
Use Debugging Tools: Utilize browser developer tools and IDE debugging features to step through your code, inspect variables, and identify the point of failure.
Simplify the Test: If possible, try to simplify the test by breaking it down into smaller, more manageable units. This can help pinpoint the source of the flakiness.
Consider Environmental Factors: Investigate if environmental factors like network connectivity, browser versions, or operating system differences might be contributing to the flakiness.
By systematically investigating and addressing these potential causes, you can effectively debug flaky tests and improve the reliability of your test suite.
17) What steps would you take to handle ElementNotInteractableException?
The ElementNotInteractableException occurs when Selenium tries to interact with an element that is present on the page but not currently interactable. This can happen due to various reasons, such as the element being hidden, disabled, or obscured by another element. Here are steps to handle this exception:
Wait for Element to be Clickable: Use an explicit wait with ExpectedConditions.elementToBeClickable() to ensure the element is interactable before attempting any action.
Java
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id(“myElement”)));
element.click();
Scroll to the Element: If the element is not in the visible viewport, scroll to it using JavaScript.
Java
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript(“arguments[0].scrollIntoView(true);”, element);
Check for Overlapping Elements: Inspect the page to see if any other element is overlapping or obscuring the target element. If so, you might need to interact with the overlapping element first (e.g., close a modal or move a slider) to make the target element interactable.
Verify Element State: Ensure that the element is not disabled or hidden. If it is, you might need to perform actions to enable or make it visible before interacting with it.
Handle iFrames: If the element is within an iFrame, switch to the iFrame before interacting with the element.
Java
driver.switchTo().frame(“frameName”);
// Interact with the element within the iFrame
driver.switchTo().defaultContent(); // Switch back to the main content
By carefully analyzing the situation and applying these techniques, you can effectively handle ElementNotInteractableException and ensure your tests run smoothly.
18) Describe how to resolve timeout issues in Selenium tests.
Timeout issues occur when a Selenium operation takes longer than the specified timeout period to complete. This can lead to exceptions like TimeoutException. Here’s how to address timeout issues:
Increase Timeout Values: If you’re encountering timeouts, consider increasing the timeout values for your waits (implicit, explicit, or Fluent Wait). This gives your tests more time to complete the operation.
Optimize Test Code: Analyze your test code for any unnecessary delays or inefficient operations. Optimize your locators, reduce unnecessary actions, and improve the overall efficiency of your test.
Investigate Network Conditions: Slow network connectivity can significantly impact test execution time. Check your network speed and stability, and consider using a more reliable network if necessary.
Analyze Application Performance: If your web application itself is slow, it can lead to timeouts. Investigate the application’s performance and identify any bottlenecks that might be causing delays.
Profile Your Tests: Use profiling tools to analyze your test execution and identify performance bottlenecks. This can help you pinpoint specific areas for optimization.
Use Asynchronous Operations: For long-running operations, consider using asynchronous techniques (if supported by your programming language and framework) to avoid blocking the main thread and prevent timeouts.
By addressing these potential causes, you can effectively resolve timeout issues and ensure your Selenium tests execute within reasonable timeframes.
19) How do you analyze and optimize test execution speed in Selenium?
Optimizing test execution speed is crucial for efficient testing and faster feedback cycles. Here are some strategies to analyze and improve your Selenium test performance:
Efficient Locators: Use the most efficient locators possible (e.g., ID, name) to identify elements. Avoid using XPath if simpler locators are available, as XPath can be slower.
Reduce Unnecessary Actions: Analyze your test scripts for any redundant or unnecessary actions. Remove any steps that don’t directly contribute to the test objective.
Optimize Waits: Use explicit waits strategically to wait for specific conditions. Avoid overusing implicit waits, as they can introduce unnecessary delays.
Page Load Strategy: Consider using the pageLoadStrategy capability to control when Selenium considers a page fully loaded. This can help optimize page load times.
Parallel Testing: Run your tests in parallel across multiple browsers or machines using Selenium Grid or cloud-based platforms. This can significantly reduce overall test execution time.
Headless Browser Execution: For certain types of tests, consider running them in headless mode (without a visible browser UI). This can improve execution speed, especially in CI/CD environments.
Profile Your Tests: Use profiling tools to identify performance bottlenecks in your test code. This can help you pinpoint areas for optimization.
By implementing these optimization techniques, you can significantly reduce your test execution time and improve the efficiency of your Selenium automation.
20) Discuss handling browser compatibility issues.
Browser compatibility issues arise when your web application behaves differently across different browsers. This can be due to variations in browser rendering engines, JavaScript implementations, or support for web standards. Here’s how to handle browser compatibility issues in Selenium:
Cross-Browser Testing: Test your web application on a variety of browsers (Chrome, Firefox, Edge, Safari) and operating systems to identify any compatibility issues.
Browser-Specific WebDriver Instances: Use browser-specific WebDriver instances (e.g., ChromeDriver, GeckoDriver) to ensure compatibility with each browser.
Cloud-Based Testing Platforms: Leverage cloud-based testing platforms like Sauce Labs or BrowserStack to access a wide range of browser and operating system combinations.
Conditional Code: Use conditional code in your test scripts to handle browser-specific behaviors. This allows you to execute different code paths based on the browser being used.
Polyfills: Use JavaScript polyfills to provide missing functionality in older browsers, ensuring consistent behavior across different browser versions.
CSS Hacks: In some cases, you might need to use CSS hacks to address browser-specific rendering inconsistencies. However, use these with caution, as they can make your code less maintainable.
By proactively addressing browser compatibility, you can ensure that your web application provides a consistent and reliable user experience across different browsers.
Apart from technical skills, soft skills like leadership and teamwork are also valued in the industry. Let’s discuss some questions related to these skills.
Selenium Interview Questions: Leadership and Team-Oriented Questions
This section assesses your leadership qualities, teamwork skills, and your ability to contribute effectively within a QA team.
21) How do you mentor junior automation testers?
Mentoring junior automation testers is crucial for their growth and development. Here’s how you can effectively mentor junior team members:
Provide Guidance and Support: Offer clear guidance on testing concepts, Selenium best practices, and framework design. Be patient and supportive in answering their questions and helping them overcome challenges.
Pair Programming: Engage in pair programming sessions to work together on test automation tasks. This allows them to learn by observing your approach and actively participate in problem-solving.
Code Reviews: Conduct regular code reviews to provide constructive feedback on their code. Focus on identifying areas for improvement, suggesting best practices, and fostering good coding habits.
Learning Resources: Share relevant learning resources like online tutorials, documentation, and books to help them expand their knowledge and skills.
Assign Challenging Tasks: Gradually assign them more challenging tasks to encourage their growth and build their confidence.
Create a Collaborative Environment: Foster a collaborative environment where they feel comfortable asking questions, sharing ideas, and seeking help.
Encourage Continuous Learning: Encourage them to stay updated with the latest testing trends and technologies through continuous learning and self-improvement.
By providing effective mentorship, you can help junior testers develop their skills, contribute effectively to the team, and grow into successful automation engineers.
22) What approach would you take to design a scalable test automation framework?
Designing a scalable test automation framework is essential for handling a growing number of tests and adapting to changing project needs. Here’s an approach to design a scalable framework:
Modularity: Break down your framework into modular components (e.g., page objects, helper classes, test data management). This promotes code reusability and makes it easier to maintain and extend the framework.
Abstraction: Abstract away implementation details from the test scripts. This makes the framework more flexible and less dependent on specific technologies or tools.
Configuration: Use configuration files to manage test data, environment settings, and other parameters. This allows you to easily modify the framework’s behavior without changing the code.
Data-Driven Testing: Implement data-driven testing to separate test data from test scripts. This makes your tests more flexible and allows you to run the same test with different data sets.
Reporting and Logging: Integrate robust reporting and logging mechanisms to provide insights into test execution and facilitate debugging.
Version Control: Use a version control system like Git to manage your codebase, track changes, and collaborate effectively with your team.
Continuous Integration: Integrate your framework with a CI/CD tool like Jenkins to automate test execution and enable faster feedback cycles.
By incorporating these design principles, you can create a scalable and maintainable test automation framework that can adapt to the evolving needs of your project.
23) Discuss how to manage large test suites efficiently.
Managing large test suites can be challenging, but with the right strategies, you can ensure efficient organization and execution. Here are some key considerations:
Organization: Organize your tests into logical groups based on functionality, modules, or test types. Use a clear and consistent naming convention for your test classes and methods.
Test Management Tools: Utilize test management tools to track test cases, organize test suites, and manage test execution. Tools like TestRail or Zephyr can help you streamline your testing process.
Prioritization: Prioritize your tests based on risk, criticality, and business impact. Focus on executing the most important tests first, especially in time-constrained situations.
Parallel Execution: Leverage parallel testing to run your tests concurrently across multiple browsers or machines. This can significantly reduce overall test execution time.
Selective Test Execution: Implement mechanisms for selective test execution, allowing you to run specific subsets of your test suite based on your needs.
Test Data Management: Efficiently manage your test data using external sources like Excel files, CSV files, or databases.
Reporting and Analysis: Use reporting tools to generate comprehensive test execution reports and analyze test results to identify trends and areas for improvement.
By implementing these strategies, you can effectively manage large test suites, ensure efficient test execution, and gain valuable insights from your testing efforts.
24) How do you ensure test coverage and quality assurance?
Ensuring comprehensive test coverage and maintaining high quality assurance are critical goals in software testing. Here’s how you can achieve these goals:
Test Planning: Develop a comprehensive test plan that outlines the scope, objectives, and testing strategies. Identify the different levels of testing (unit, integration, system) and the types of testing (functional, performance, security) required.
Requirements Analysis: Thoroughly analyze the requirements to understand the expected behavior of the application. This helps you design effective test cases that cover all functionalities.
Test Design Techniques: Utilize various test design techniques like equivalence partitioning boundary value analysis, and state transition testing to design effective test cases.
Code Reviews: Conduct regular code reviews to identify potential defects and ensure adherence to coding standards. This helps catch errors early in the development cycle.
Static Analysis: Use static analysis tools to analyze your code for potential issues like code smells, security vulnerabilities, and performance bottlenecks.
Test Automation: Automate your tests to increase test coverage and execution speed. This allows you to run tests more frequently and catch regressions early.
Continuous Integration: Integrate your testing process with your CI/CD pipeline to ensure that tests are run automatically with every code change.
Monitoring and Feedback: Continuously monitor your application in production and gather feedback from users to identify areas for improvement and address potential issues.
By implementing these practices, you can ensure that your software is thoroughly tested, meets quality standards, and provides a positive user experience.
25) How do you handle conflicts within a testing team?
Conflicts are inevitable in any team environment, and it’s important to handle them constructively to maintain a healthy and productive team dynamic. Here’s how to approach conflicts within a testing team:
Open Communication: Encourage open and honest communication among team members. Create a safe space where individuals feel comfortable 1 expressing their concerns and perspectives.
Active Listening: When a conflict arises, actively listen to all parties involved. Try to understand their viewpoints and the root cause of the conflict.
Focus on the Issue: Keep the focus on the issue at hand, rather than making it personal. Avoid blaming or attacking individuals.
Find Common Ground: Look for areas of agreement and common goals. This helps shift the focus towards collaboration and finding solutions.
Brainstorm Solutions: Facilitate a brainstorming session to generate potential solutions to the conflict. Encourage everyone to contribute ideas.
Compromise and Collaboration: Encourage team members to be willing to compromise and collaborate to find a solution that works for everyone.
Mediation: If necessary, involve a neutral third party to mediate the conflict and help facilitate a resolution.
Follow Up: After a resolution is reached, follow up to ensure that the agreed-upon solution is being implemented and that the conflict has been truly resolved.
By addressing conflicts in a constructive and collaborative manner, you can foster a positive team environment and ensure that everyone feels valued and respected.
In addition to the core concepts and technical skills, you should also be aware of the latest trends and emerging technologies in the automation field.
Selenium Interview Questions: Miscellaneous and Emerging Trends
This section explores broader topics related to Selenium and emerging trends in the testing field, demonstrating your awareness of industry advancements and your ability to adapt to new technologies.
26) How would you implement Selenium with AI/ML testing tools?
The integration of Artificial Intelligence (AI) and Machine Learning (ML) with Selenium is transforming the way we approach test automation. Here’s how you can leverage AI/ML tools with Selenium:
Visual Testing: AI-powered visual testing tools like Applitools or Percy can analyze screenshots of your web pages and detect visual regressions, even subtle UI changes that might be missed by traditional Selenium tests.
Self-Healing Tests: AI can be used to create self-healing tests that automatically adapt to changes in the application’s UI. This reduces test maintenance and improves test stability.
Test Case Generation: AI/ML algorithms can analyze application code and user behavior to automatically generate test cases, improving test coverage and efficiency.
Test Data Generation: AI can be used to generate realistic and diverse test data, enhancing the effectiveness of your data-driven testing.
Log Analysis: ML algorithms can analyze test logs to identify patterns, predict failures, and provide insights into test effectiveness.
By integrating Selenium with AI/ML testing tools, you can enhance your automation efforts, improve test coverage, and reduce test maintenance.
27) What’s your experience with mobile testing using Selenium?
While Selenium is primarily designed for web application testing, it can be used in conjunction with Appium for mobile testing. Appium is an open-source framework that uses the WebDriver protocol to automate mobile apps on iOS and Android platforms.
To use Selenium with Appium, you would typically:
Set up Appium: Install Appium and configure it to connect to your mobile devices or emulators.
Use Appium Client Libraries: Use Appium client libraries (available for various programming languages) in your Selenium tests to interact with mobile elements and perform actions on the mobile app.
Define Desired Capabilities: Define the desired capabilities for your mobile device or emulator, including platform name, platform version, device name, and app path.
Write Selenium Tests: Write your Selenium tests using the Appium client libraries to automate actions on the mobile app.
While Appium is the preferred choice for dedicated mobile testing, understanding how to use Selenium in conjunction with Appium can be valuable for scenarios where you need to integrate web and mobile testing.
28) How do you handle testing for SPAs (Single Page Applications)?
Single Page Applications (SPAs) present unique challenges for Selenium testing due to their dynamic nature and heavy reliance on JavaScript. Here’s how to handle SPA testing:
Explicit Waits: Use explicit waits extensively to ensure that elements are loaded and interactable before interacting with them. SPAs often update the DOM dynamically, so waiting for specific conditions is crucial.
Asynchronous JavaScript: Be mindful of asynchronous JavaScript operations. Use explicit waits to ensure that these operations have completed before proceeding with your test.
Network Monitoring: Use browser developer tools or network monitoring tools to understand the network requests and responses in your SPA. This can help you identify potential timing issues or delays.
JavaScript Executors: Use the JavascriptExecutor to execute JavaScript code within the browser. This can be helpful for interacting with elements that are not directly accessible through Selenium or for performing actions that require JavaScript.
Headless Browser Testing: Consider running your SPA tests in headless mode to improve execution speed, especially in CI/CD environments.
By understanding the specific challenges of SPA testing and applying these techniques, you can ensure that your Selenium tests effectively cover the functionality of your SPA.
29) Explain the role of Selenium in Agile and DevOps environments.
Selenium plays a vital role in Agile and DevOps environments by enabling test automation, which is crucial for achieving faster feedback cycles and continuous delivery. Here’s how Selenium contributes:
Automated Regression Testing: Selenium allows you to automate regression tests, ensuring that new code changes don’t break existing functionality. This is essential for frequent releases in Agile and DevOps.
Continuous Integration: Selenium integrates seamlessly with CI/CD tools like Jenkins, enabling automated test execution as part of the build process. This ensures that code changes are continuously tested and that any issues are identified early.
Faster Feedback Loops: Automated tests provide rapid feedback on the quality of the code, allowing developers to address issues quickly and maintain a fast development pace.
Increased Test Coverage: Automation allows you to run a larger number of tests more frequently, increasing test coverage and improving the overall quality of the application.
Reduced Manual Effort: By automating repetitive testing tasks, Selenium frees up testers to focus on more exploratory or strategic testing activities.
By effectively utilizing Selenium in Agile and DevOps, you can accelerate your testing process, improve code quality, and contribute to a more efficient software development lifecycle.
30) Discuss future trends in Selenium automation.
Selenium continues to evolve, and staying ahead of the curve requires awareness of emerging trends:
AI-Powered Automation: The integration of AI and ML with Selenium is expected to deepen, with more intelligent and self-healing tests becoming prevalent.
Codeless Automation: Codeless automation tools are gaining popularity, allowing testers to create automated tests without writing code. This trend is likely to continue, making Selenium more accessible to a wider audience.
Mobile Testing Advancements: While Appium remains the primary choice for mobile testing, Selenium might see further integration with mobile testing tools and frameworks.
Cross-Browser Compatibility Focus: As new browsers and browser versions emerge, ensuring cross-browser compatibility will remain a key focus for Selenium.
Integration with Cloud and DevOps: Selenium’s integration with cloud-based testing platforms and DevOps tools is expected to strengthen, further streamlining the testing process.
By staying informed about these trends and continuously learning, you can ensure your Selenium skills remain relevant and valuable in the ever-evolving world of software testing.
To ace your Selenium interview, effective preparation is key. Let’s discuss some valuable tips to help you prepare.
Preparation Tips for Selenium Interviews
To prepare for your Selenium interview, practice coding challenges, review Selenium documentation, and work on real-world automation projects.
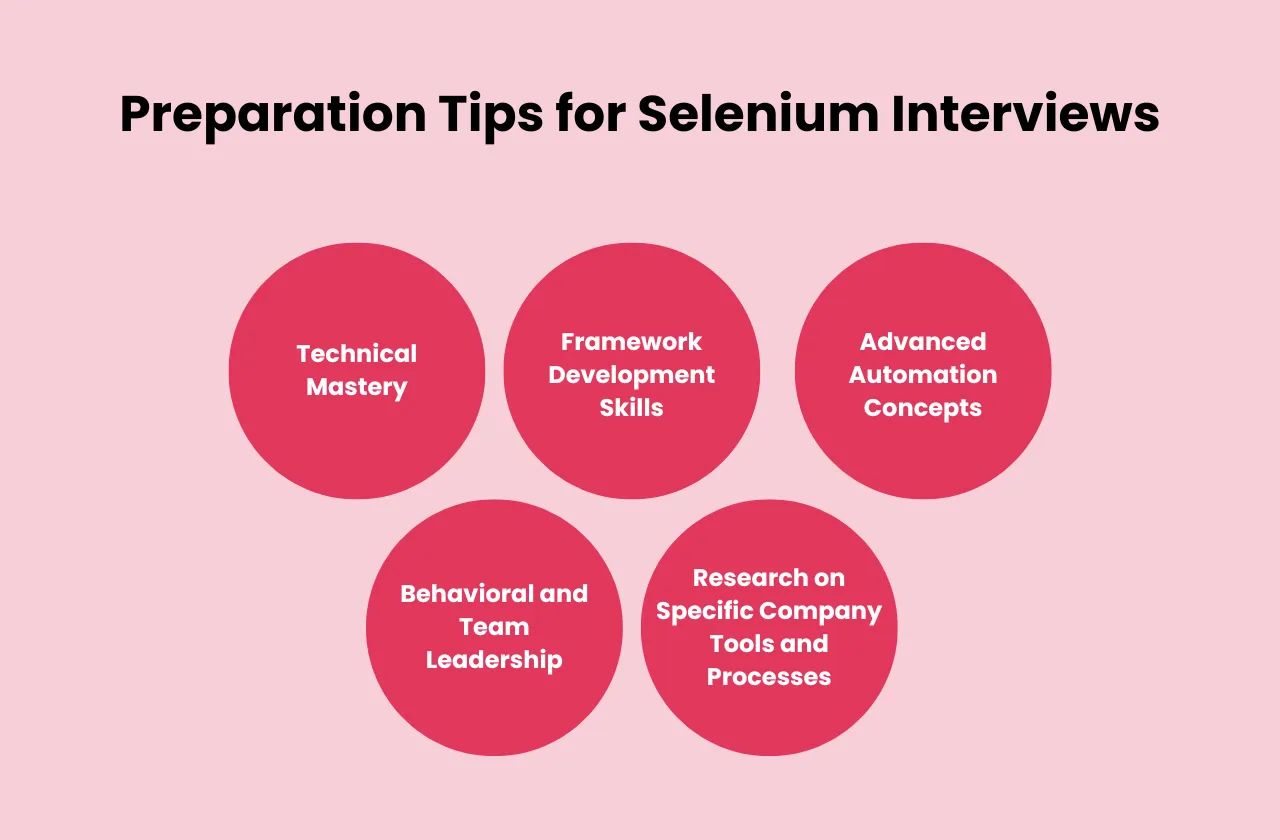
A) Technical Mastery
Deep Dive into Selenium WebDriver: Go beyond the basics. Understand the intricacies of WebDriver, including different locator strategies, handling various web elements (dropdowns, alerts, iframes), and managing browser windows and tabs.
Master Selenium Grid and Parallel Testing: Learn how to set up and configure Selenium Grid for distributed testing. Understand the benefits of parallel testing and how to implement it effectively.
B) Framework Development Skills
Practice Designing Custom Frameworks: Don’t just rely on existing frameworks. Practice building your own frameworks from scratch using TestNG, JUnit, or other testing frameworks. This demonstrates your ability to design robust and maintainable automation solutions.
Gain Proficiency in CI/CD Tools: Familiarize yourself with popular CI/CD tools like Jenkins, GitLab CI/CD, or Azure DevOps. Understand how to integrate Selenium tests into your CI/CD pipeline for automated test execution.
C) Advanced Automation Concepts
Handle Complex Scenarios: Practice handling challenging scenarios like dynamic elements, AJAX calls, shadow DOM elements, and multi-tab interactions. This showcases your ability to tackle real-world automation challenges.
Integrate Selenium with Other Tools: Expand your skillset by learning how to integrate Selenium with other testing tools like Appium for mobile testing, REST Assured for API testing, or JMeter for performance testing.
D) Behavioral and Team Leadership
Emphasize Communication and Collaboration: Be prepared to discuss your experiences working in a team environment, your communication style, and your approach to conflict resolution.
Highlight Leadership Qualities: If you have leadership experience, showcase your ability to mentor, guide, and motivate team members. Demonstrate your understanding of effective team dynamics and your ability to contribute to a positive work environment.
E) Research on Specific Company Tools and Processes
Understand the Company’s Testing Approach: Research the company you’re interviewing with to understand their testing methodologies, tools, and technologies. Tailor your answers to align with their specific needs and practices.
Prepare Questions to Ask: Asking insightful questions demonstrates your interest in the company and the role. Prepare questions about their testing processes, challenges they face, or their future plans for automation.
By following these tips and staying updated with the latest trends, you can confidently face your Selenium interview and land your dream job.
Conclusion
This comprehensive guide has equipped you with the knowledge and strategies to confidently tackle your Selenium interviews. By mastering the core concepts, advanced techniques, and integration with other tools, you can demonstrate your expertise and impress potential employers. Remember to practice your answers, highlight your problem-solving skills, and showcase your passion for software testing. With thorough preparation and a confident attitude, you’ll be well on your way to landing your dream job in the exciting field of Selenium automation.