Salesforce Apex is a critical skill for developers working in the Salesforce ecosystem. Apex, as Salesforce’s proprietary programming language, is used to develop custom applications, automate complex business processes, and handle integrations with external systems. For job seekers aiming for Salesforce development roles, a deep understanding of Apex is essential. However, facing Apex interview questions can often be challenging, as they test both theoretical knowledge and practical skills.
This article aims to prepare you for Apex interviews by exploring top questions and answers in detail. Whether you’re a beginner or an experienced developer, mastering these concepts will enable you to perform confidently in interviews. This guide covers all major areas of Apex, from basic concepts to advanced topics, including tips on how to ace your interview.
Salesforce Apex Interview Questions: Basic Apex Concepts
Before we get into the nitty-gritty of Salesforce Apex, let’s make sure you have a solid understanding of the basic concepts. In this section, we’ll cover topics like classes, objects, methods, and variables.
Question 1: What is Salesforce Apex?
Salesforce Apex is an object-oriented programming language used to develop custom business logic on the Salesforce platform. It’s strongly typed, meaning variables must be declared with a specific data type, and it’s compiled and executed natively within the Salesforce environment.
Apex enables developers to create everything from simple business logic, such as automating workflows and processes, to building complex applications and integrations with external systems. It is modelled after Java and includes built-in support for database transactions, meaning developers can use Apex to interact directly with Salesforce data through SOQL (Salesforce Object Query Language) and SOSL (Salesforce Object Search Language).
Apex operates entirely on the Salesforce platform and is designed to run efficiently in a multitenant environment where multiple clients share the same infrastructure. The language provides built-in security and sharing rules, helping developers maintain data security and access control without requiring additional code.
Key features of Salesforce Apex include:
- Tight integration with Salesforce: Apex allows seamless interaction with Salesforce objects and data.
- Triggered execution: Apex code can be triggered by database events like insertions or updates.
- Asynchronous processing: Apex supports asynchronous operations, allowing developers to execute tasks outside the context of the current transaction.
- Robust error handling: Apex includes error-handling mechanisms like try-catch blocks for managing exceptions.
- Batch processing: Apex allows developers to process large volumes of data asynchronously using batch Apex classes.
Question 2: What are the different types of Apex code?
Salesforce Apex supports several types of code structures that serve different purposes. Understanding these types is crucial for writing efficient, maintainable, and scalable code. Below are the main types of Apex code:
Trigger: Triggers are pieces of Apex code that execute automatically in response to specific events, such as when records are inserted, updated, or deleted. Triggers are used to enforce business rules, update related records, and perform other automation tasks.
Example of a basic trigger:
apex
trigger AccountTrigger on Account (before insert) {
for (Account acc : Trigger.new) {
acc.Name = acc.Name + ‘ – Updated’;
}
}
Visualforce Controller: Visualforce controllers handle interactions between the Visualforce page and the database. There are two types of controllers:
Standard controller: Provided by Salesforce, these controllers manage standard Salesforce objects and allow basic CRUD operations.
Custom controller: Written in Apex, these controllers allow developers to implement custom business logic and extend or override the functionality of standard controllers.
Example of a custom controller:
apex
public class MyCustomController {
public Account acc {get; set;}
public MyCustomController() {
acc = [SELECT Name, Industry FROM Account WHERE Id = :ApexPages.currentPage().getParameters().get(‘id’)];
}
}
Batch Apex: Batch Apex is used to handle large-scale data operations. When dealing with thousands or even millions of records, Batch Apex processes data in manageable chunks (or batches), reducing the risk of hitting governor limits. Batch Apex jobs are typically executed asynchronously.
Future Methods: Future methods allow developers to execute code asynchronously, meaning that it doesn’t have to run in real-time and can be processed later. Future methods are typically used when making callouts to external web services or when you need to perform a long-running operation that doesn’t need to be completed immediately.
Example of a future method:
apex
@future
public static void makeCallout() {
// Asynchronous logic here
}
Question 3: Explain the difference between static and instance methods.
In Apex, methods can either be static or instance-based, and knowing when to use each type is key to writing efficient code.
Static methods: These are methods that belong to the class itself, not to any specific instance of the class. You can call a static method without creating an instance of the class. Static methods are typically used for utility methods or when the method’s logic doesn’t depend on an object’s state.
Example of a static method:
apex
public class Utility {
public static Integer addNumbers(Integer a, Integer b) {
return a + b;
}
}
Here, addNumbers is a static method, so you can call it like this: Utility.addNumbers(5, 10);.
Instance methods: These methods require an instance of the class to be created before you can call them. Instance methods usually interact with instance variables, meaning they rely on the state of the particular object they are associated with.
Example of an instance method:
apex
public class Calculator {
public Integer num1;
public Integer num2;
public Integer add() {
return num1 + num2;
}
}
Here, you would need to create an instance of Calculator to use the add() method:
apex
Calculator calc = new Calculator();
calc.num1 = 5;
calc.num2 = 10;
Integer result = calc.add();
Question 4: What is a governor limit in Apex, and why are they important?
Salesforce operates in a multitenant environment, which means multiple organisations (tenants) share the same instance of Salesforce. To ensure that no single tenant consumes excessive resources and impacts others, Salesforce enforces governor limits. These limits are restrictions on the resources a single transaction can consume, and they exist to prevent inefficiencies and ensure fair resource allocation.
Some of the most common governor limits include:
- SOQL queries: A maximum of 100 SOQL queries are allowed per transaction. If you exceed this limit, your transaction will fail.
- DML statements: You are allowed a maximum of 150 DML operations per transaction. DML operations include operations like INSERT, UPDATE, DELETE, etc.
- CPU time: The maximum allowed CPU time per transaction is 10,000 milliseconds. Transactions that exceed this time limit will be terminated.
- Heap size: Apex limits the amount of memory you can use in a transaction to store data. The maximum heap size is 6 MB for synchronous Apex and 12 MB for asynchronous Apex.
Governor limits are important because exceeding them causes the entire transaction to fail. Developers need to be mindful of these limits when writing code and ensure that their logic handles bulk records efficiently to avoid errors.
Best Practices to Avoid Exceeding Governor Limits:
- Bulkify your code: Write your code to handle multiple records at once rather than processing each record individually.
- Use collections: Group records into collections such as lists or sets to process them in bulk.
- Avoid SOQL queries in loops: Querying the database inside a loop can quickly lead to exceeding the query limit. Instead, retrieve all necessary data outside the loop.
- Use batch Apex for large data operations: For operations involving thousands of records, use Batch Apex to process data in manageable chunks.
Now that you have a good grasp of the fundamentals, let’s move on to the next section and learn about Apex syntax and data types.
Salesforce Apex Interview Questions: Apex Syntax and Data Types
Understanding Apex syntax and data types is essential for writing effective Apex code. In this section, we’ll discuss topics like operators, control flow statements, and data types.
Question 5: Describe the basic syntax of an Apex class and method.
Apex is a statically typed language, meaning every variable and method must be declared with a type. Below is the basic syntax for defining a class and a method in Apex.
Class: A class in Apex is a blueprint from which objects are created. A class defines the properties (variables) and methods (functions) that an object can have.
Syntax of a class:
apex
public class MyClass {
public Integer myVariable; // Instance variable
// Method to print “Hello, World!”
public void printMessage() {
System.debug(‘Hello, World!’);
}
}
Method: A method in Apex is a function that performs a specific task. A method can have input parameters and return a value.
Syntax of a method:
apex
public Integer addNumbers(Integer a, Integer b) {
return a + b;
}
In this example, addNumbers is a method that takes two integers as input, adds them together, and returns the result.
Question 6: What are the different data types in Apex?
Apex supports a variety of data types, both primitive and complex. Understanding these types is essential for writing effective Apex code.
Primitive Data Types:
- String: Represents a sequence of characters.
- Integer: Represents a whole number without a decimal.
- Boolean: Represents a true/false value.
- Decimal: Represents a number that includes a decimal point.
- Date: Represents a date (without time).
- DateTime: Represents both a date and time.
- Time: Represents a time of day (without a date).
Example:
apex
String name = ‘John Doe’;
Integer age = 30;
Boolean isActive = true;
Complex Data Types:
- SObject: Represents a Salesforce object, such as Account, Contact, or any custom object. You can retrieve or manipulate Salesforce data using SObjects.
- Lists: An ordered collection of elements, where each element can be accessed by its index.
- Sets: An unordered collection of unique elements, useful for ensuring no duplicates.
- Maps: A collection of key-value pairs, where each key maps to a specific value.
Example of SObject:
apex
Account acc = new Account();
acc.Name = ‘Acme Corporation’;
insert acc;
Question 7: Explain the concept of a List and Set in Apex.
List: A List in Apex is an ordered collection of elements that allows duplicates. Each element in the list can be accessed using its index, starting from 0. Lists are useful when the order of elements matters, or when you may have duplicate values.
Common operations on a List include:
- Adding an element: list.add(element)
- Removing an element: list.remove(index)
- Retrieving an element: list.get(index)
Example:
apex
List<String> names = new List<String>();
names.add(‘John’);
names.add(‘Jane’);
names.add(‘John’); // Duplicate is allowed
System.debug(names.get(0)); // Outputs ‘John’
Set: A Set in Apex is an unordered collection that does not allow duplicate values. Sets are useful when you need to ensure uniqueness, and the order of elements is not important.
Common operations on a Set include:
- Adding an element: set.add(element)
- Removing an element: set.remove(element)
- Checking if an element exists: set.contains(element)
Example:
apex
Set<String> uniqueNames = new Set<String>();
uniqueNames.add(‘John’);
uniqueNames.add(‘Jane’);
uniqueNames.add(‘John’); // Duplicate will be ignored
System.debug(uniqueNames.contains(‘John’)); // Outputs ‘true’
With a strong foundation in syntax and data types, let’s explore how to use triggers and DML operations in Salesforce Apex.
Salesforce Apex Interview Questions: Triggers and DML Operations
Triggers and DML operations are powerful tools for automating tasks in Salesforce. In this section, we’ll discuss how to create and use triggers, as well as how to perform DML operations.
Question 8: What is a trigger, and how does it work?
A trigger is a piece of Apex code that is executed automatically before or after specific events on a Salesforce object, such as insertions, updates, deletions, or undeletions. Triggers are used to enforce business rules, automate processes, and handle complex scenarios that cannot be achieved through declarative tools like Process Builder or Flow.
Triggers can operate in two main contexts:
Before triggers: These triggers are executed before the record is saved to the database. Before triggers are typically used to validate or modify data before the DML operation occurs.
After triggers: These triggers are executed after the record has been saved to the database. After triggers are commonly used to update related records or perform operations that require the record’s ID.
Example of a before-insert trigger:
apex
trigger AccountBeforeInsert on Account (before insert) {
for (Account acc : Trigger.new) {
acc.Name = acc.Name + ‘ – Verified’;
}
}
Question 9: Explain the different types of triggers.
There are six main types of triggers in Salesforce, based on the event that triggers them:
- Before Insert: Executes before a record is inserted into the database.
- Before Update: Executes before a record is updated in the database.
- Before Delete: Executes before a record is deleted from the database.
- After Insert: Executes after a record is inserted into the database.
- After Update: Executes after a record is updated in the database.
- After Delete: Executes after a record is deleted from the database.
Each type of trigger is used based on the specific operation you need to perform. Before triggers are usually used for data validation or manipulation, while after triggers are used when you need to perform actions that require the record’s ID or are dependent on the success of the DML operation.
Question 10: What are DML operations in Apex?
DML (Data Manipulation Language) operations in Apex allow developers to perform the following operations on Salesforce records:
INSERT: Adds new records to the Salesforce database.
apex
Account acc = new Account(Name=’Acme Inc’);
insert acc;
UPDATE: Modifies existing records in the Salesforce database.
apex
Account acc = [SELECT Id, Name FROM Account WHERE Name = ‘Acme Inc’];
acc.Name = ‘Acme Corporation’;
update acc;
DELETE: Removes records from the Salesforce database.
apex
Account acc = [SELECT Id FROM Account WHERE Name = ‘Acme Corporation’];
delete acc;
UNDELETE: Recovers records that were previously deleted from the Recycle Bin.
apex
List<Account> accounts = [SELECT Id FROM Account WHERE IsDeleted = true];
undelete accounts;
MERGE: Combines up to three records of the same type into one record. Merge is used to consolidate duplicate records.
apex
Account masterAccount = [SELECT Id FROM Account WHERE Name = ‘Acme Corporation’];
Account duplicateAccount = [SELECT Id FROM Account WHERE Name = ‘Acme Ltd’];
merge masterAccount duplicateAccount;
Question 11: How can you prevent infinite loops in triggers?
Infinite loops in triggers occur when a trigger performs an update on a record that causes the same trigger to execute again, leading to an endless cycle. To prevent this, you can use several techniques:
Static variables: Use a static variable to track whether the trigger has already executed. Static variables retain their value throughout the execution of a single transaction, allowing you to prevent the trigger from running more than once.
Example:
apex
public class TriggerHelper {
public static Boolean isFirstRun = true;
}
trigger AccountTrigger on Account (before update) {
if (TriggerHelper.isFirstRun) {
TriggerHelper.isFirstRun = false;
// Trigger logic here
}
}
Conditional checks: Add conditional checks to ensure that the trigger only executes when specific conditions are met. For example, only run the trigger if a certain field value has changed.
Example:
apex
trigger AccountTrigger on Account (before update) {
for (Account acc : Trigger.new) {
if (acc.Name != Trigger.oldMap.get(acc.Id).Name) {
// Perform update logic
}
}
}
Trigger frameworks: Use trigger frameworks to control the execution flow and manage recursion. Many frameworks provide built-in mechanisms for avoiding infinite loops.
Now that you know how to use triggers and DML operations, let’s move on to the next section and learn about Visualforce and controllers.
Salesforce Apex Interview Questions: Visualforce and Controllers
Visualforce is a powerful tool for creating custom user interfaces in Salesforce. In this section, we’ll discuss how to create Visualforce pages and controllers.
Question 12: What is Visualforce, and how is it used with Apex?
Visualforce is a framework that allows developers to create custom user interfaces for Salesforce applications. It consists of a tag-based markup language (similar to HTML) and integrates seamlessly with Apex to create dynamic web pages. Visualforce pages can either use standard Salesforce controllers or custom controllers written in Apex.
Visualforce is commonly used when standard Salesforce layouts and components are not sufficient for a business’s needs. It provides greater flexibility and control over the look and behaviour of Salesforce pages.
Example of a simple Visualforce page:
html
<apex:page standardController=”Account”>
<apex:form>
<apex:pageBlock title=”Account Information”>
<apex:pageBlockSection>
<apex:outputField value=”{!Account.Name}” />
<apex:outputField value=”{!Account.Industry}” />
</apex:pageBlockSection>
</apex:pageBlock>
</apex:form>
</apex:page>
Question 13: Explain the difference between a standard controller and a custom controller.
Standard controller: A standard controller is provided by Salesforce and gives basic functionality for working with a specific object, such as viewing, editing, and saving records. It does not require custom code and is useful for simple use cases.
Example of using a standard controller in Visualforce:
html
<apex:page standardController=”Account”>
<apex:form>
<apex:outputField value=”{!Account.Name}” />
</apex:form>
</apex:page>
Custom controller: A custom controller is written in Apex and allows for more complex logic and interactions with the Salesforce database. Custom controllers are used when the standard controller does not meet the business requirements or when you need to implement specific logic.
Example of a custom controller:
apex
public class MyAccountController {
public Account acc {get; set;}
public MyAccountController() {
acc = [SELECT Name FROM Account WHERE Id = :ApexPages.currentPage().getParameters().get(‘id’)];
}
}
In the Visualforce page:
html
<apex:page controller=”MyAccountController”>
<apex:outputField value=”{!acc.Name}” />
</apex:page>
Question 14: What is a component in Visualforce?
A component in Visualforce is a reusable piece of the user interface, such as an input field, button, or table. Components make it easy to build complex interfaces by encapsulating commonly used functionality into a single, reusable unit. Visualforce provides a wide range of built-in components for creating forms, tables, and other elements of the user interface.
Common Visualforce components include:
- <apex:inputField>: A field that allows the user to input data.
- <apex:commandButton>: A button that triggers an action.
- <apex:pageBlockTable>: A table that displays data from a list or collection.
Example:
html
<apex:page standardController=”Account”>
<apex:form>
<apex:inputField value=”{!Account.Name}” />
<apex:commandButton action=”{!save}” value=”Save” />
</apex:form>
</apex:page>
Question 15: How do you pass parameters to a Visualforce page?
You can pass parameters to a Visualforce page using the URL. These parameters can then be accessed in the controller using the ApexPages.currentPage().getParameters() method.
For example, if you pass a parameter in the URL like this:
bash
/apex/MyPage?id=0017F00000abc123
You can retrieve this parameter in the controller:
apex
public class ParameterController {
public String recordId;
public ParameterController() {
recordId = ApexPages.currentPage().getParameters().get(‘id’);
}
}
In this example, the id parameter is retrieved from the URL and stored in the recordId variable.
Visualforce and controllers are essential for building custom user interfaces, but what about large-scale data processing? Let’s explore Batch Apex in the next section.
Salesforce Apex Interview Questions: Batch Apex
Batch Apex is a powerful tool for processing large datasets in Salesforce. In this section, we’ll discuss how to create and use Batch Apex jobs.
Question 16: What is Batch Apex, and when is it used?
Batch Apex is used to process large volumes of records asynchronously in Salesforce. It allows developers to break down large data operations into manageable chunks, each processed separately, which helps avoid hitting governor limits like the SOQL query limit or CPU time limit.
Batch Apex is commonly used in scenarios such as:
- Processing large datasets that exceed governor limits.
- Performing complex calculations or updates on thousands or millions of records.
- Integrating with external systems where data needs to be processed in bulk.
- Batch Apex operates in three main phases: start, execute, and finish.
Question 17: Explain the three methods in a Batch Apex job (start, execute, finish).
Start: This method is called at the beginning of the batch job and is used to define the scope of data that will be processed. The start method returns a Database.QueryLocator or an Iterable of the records to be processed.
Example:
apex
global Database.QueryLocator start(Database.BatchableContext BC) {
return Database.getQueryLocator([SELECT Id, Name FROM Account]);
}
Execute: This method is called once for each batch of records that are processed. The logic for processing each batch is written inside this method.
Example:
apex
global void execute(Database.BatchableContext BC, List<Account> scope) {
for (Account acc : scope) {
acc.Name = acc.Name + ‘ – Processed’;
}
update scope;
}
Finish: This method is called after all batches have been processed. It is typically used for post-processing, such as sending confirmation emails or performing final cleanup.
Example:
apex
global void finish(Database.BatchableContext BC) {
// Final logic after batch processing
}
Question 18: What is a scope in Batch Apex?
Scope refers to the number of records processed in each batch during a Batch Apex job. The default scope is 200 records, but it can be customised by the developer. The execute method will be called once for each batch, with the number of records specified by the scope.
Example of setting a custom scope:
apex
MyBatchClass batchJob = new MyBatchClass();
Database.executeBatch(batchJob, 100); // Set scope to 100
Batch Apex is a great tool for handling large-scale data processing, but what about executing code asynchronously? Let’s learn about Future Methods in the next section.
Salesforce Apex Interview Questions: Future Methods
Future Methods allow you to execute code asynchronously in Salesforce. In this section, we’ll discuss how to create and use Future Methods.
Question 19: What is a future method, and how is it used for asynchronous processing?
Future methods in Apex allow developers to execute logic asynchronously, meaning the method will be executed at a later time rather than immediately. This is particularly useful for long-running operations or tasks that need to make a callout to an external web service, which cannot be done synchronously.
To define a future method, you use the @future annotation.
Example of a future method:
apex
@future
public static void callExternalService() {
// Callout logic here
}
Future methods are commonly used in scenarios where you need to:
- Make a web service callout to an external system.
- Process data outside of the transaction context (e.g., after the main transaction is complete).
- Perform long-running calculations or updates without affecting the user experience.
Question 20: What are the limitations of future methods?
While future methods are useful for asynchronous processing, they do come with some limitations:
- No return values: Future methods cannot return a value to the caller. They are designed to run in the background and cannot communicate directly with the calling code.
- Limited number of future calls: You can only have up to 50 future method invocations per transaction. This limit ensures that asynchronous processing does not overload the system.
- Cannot call other future methods: A future method cannot call another future method, batch job, or scheduled Apex job.
- No guarantee of immediate execution: Future methods are queued for execution, but Salesforce does not guarantee that they will run immediately. There can be delays based on system load and resource availability.
Future Methods are useful for executing code asynchronously, but what happens when things go wrong? Let’s explore error handling and debugging in the next section.
Salesforce Apex Interview Questions: Error Handling and Debugging
Errors are a fact of life in programming. In this section, we’ll discuss how to handle errors and debug your Apex code.
Question 21: How do you handle errors in Apex using try-catch blocks?
Error handling in Apex is done using the try-catch construct. This allows you to write code that attempts to execute a block of logic (try block) and catches any exceptions that occur during execution (catch block). By handling errors gracefully, you can prevent runtime failures and provide useful feedback to the user or system.
Example:
apex
try {
// Code that may throw an exception
Account acc = new Account(Name=null);
insert acc; // This will cause a validation error
} catch (DmlException e) {
System.debug(‘Error: ‘ + e.getMessage());
}
In this example, the DmlException is caught in the catch block, and the error message is logged using System.debug().
Question 22: What is a custom exception, and when is it used?
A custom exception is a user-defined exception that extends the Exception class in Apex. Custom exceptions are used to handle specific error conditions in your application that are not covered by the standard Salesforce exceptions.
To create a custom exception, you define a new class that extends Exception:
apex
public class MyCustomException extends Exception {}
You can then throw this exception in your code when a specific condition occurs:
apex
if (someConditionFails) {
throw new MyCustomException(‘Custom error occurred.’);
}
Custom exceptions are useful for creating more meaningful and specific error messages, improving the debugging and error-handling process.
Question 23: Describe the different debugging tools available in Salesforce.
Salesforce provides several tools for debugging Apex code:
Debug logs: Debug logs capture detailed information about code execution, including executed lines of code, database queries, DML operations, and exceptions. Developers can view debug logs in Salesforce Setup by navigating to “Debug Logs” and selecting the relevant log entry.
System.debug(): This method is used to log custom messages or variables during code execution. It helps developers inspect variable values and understand the flow of the program.
Apex Test Runner: The Test Runner allows developers to run unit tests on their Apex classes and methods, ensuring that their code behaves as expected. Test results are displayed with detailed information about any failed tests and the reasons for failure.
Developer Console: The Developer Console is a powerful tool in Salesforce that provides features like real-time debugging, code execution tracking, and log monitoring. It allows developers to write, run, and debug Apex code directly within the Salesforce environment.
Now that you know how to handle errors and debug your code, let’s discuss some best practices related questions for writing efficient and maintainable Apex code.
Salesforce Apex Interview Questions: Best Practices and Performance Optimization
Writing clean, efficient, and maintainable Apex code is essential. In this section, we’ll discuss some best practices related questions for writing high-quality Apex code.
Question 24: What are some best practices for writing efficient Apex code?
Writing efficient Apex code is crucial for maintaining system performance and ensuring that your applications can handle large data volumes without exceeding governor limits. Below are some best practices for writing efficient Apex code:
Bulkification: Write code that processes multiple records at once, rather than operating on a single record. This is particularly important when working with triggers, batch jobs, and future methods.
Use collections: Use lists, sets, and maps to group records together and perform operations in bulk. For example, instead of inserting or updating records one at a time, add them to a list and perform a single DML operation on the entire list.
Avoid SOQL queries in loops: Querying the database inside a loop can quickly lead to exceeding the SOQL query limit. Instead, retrieve all necessary data outside the loop.
Bad practice:
apex
for (Account acc : accounts) {
List<Contact> contacts = [SELECT Id FROM Contact WHERE AccountId = :acc.Id];
}
Good practice:
apex
List<Contact> contacts = [SELECT Id FROM Contact WHERE AccountId IN :accountIds];
Limit the number of DML statements: Similar to SOQL queries, DML operations (insert, update, delete) should be performed in bulk to avoid hitting governor limits. Instead of performing multiple individual DML operations, group records into collections and perform a single operation.
Use @future and Batch Apex for long-running processes: For tasks that involve large datasets or long-running operations, use asynchronous processing methods like future methods or batch Apex.
Question 25: How can you optimise query performance in Apex?
Optimising query performance is critical for ensuring that your Apex code runs efficiently, especially when dealing with large datasets. Here are some techniques for optimising query performance:
Select only the fields you need: Avoid using SELECT * in your SOQL queries. Instead, specify only the fields you need to reduce the amount of data retrieved from the database.
apex
// Avoid this
List<Account> accounts = [SELECT * FROM Account];
// Do this instead
List<Account> accounts = [SELECT Id, Name FROM Account];
Use indexed fields: Indexed fields improve query performance by allowing Salesforce to quickly locate records. Commonly indexed fields include Id, Name, and OwnerId, but custom fields can also be indexed.
Limit the number of records retrieved: Use the LIMIT keyword to restrict the number of records returned by your query. This is especially useful when you only need a small subset of records.
apex
List<Account> accounts = [SELECT Id, Name FROM Account LIMIT 100];
Use selective filters: Apply filters that significantly reduce the number of records retrieved by the query. Avoid using broad or non-selective filters that result in retrieving large amounts of data.
Use query plan and query optimizer: Salesforce provides a Query Plan tool in the Developer Console to analyse and optimise complex queries. Use this tool to understand how Salesforce is executing your query and identify areas for improvement.
Question 26: Explain the concept of bulkification in Apex.
Bulkification refers to the process of writing code that can handle multiple records at once, rather than processing a single record at a time. This is a fundamental concept in Apex because Salesforce enforces strict governor limits on resources like SOQL queries and DML operations.
By bulkifying your code, you can avoid hitting these limits and ensure that your logic can handle large datasets efficiently. Bulkification is especially important in triggers, where operations on multiple records can easily exceed governor limits if not properly managed.
Example of bulkified code:
apex
List<Account> accountsToUpdate = new List<Account>();
for (Account acc : Trigger.new) {
acc.Name = acc.Name + ‘ – Updated’;
accountsToUpdate.add(acc);
}
update accountsToUpdate;
In this example, the code processes all accounts in the trigger at once and performs a single update operation, rather than updating each account individually.
We’ve covered a lot of ground in this article, but there are still more advanced topics to explore. Let’s dive into some advanced topics in the next section.
Salesforce Apex Interview Questions: Advanced Topics
In this section, we’ll explore some more advanced topics in Salesforce Apex, such as Apex REST APIs, Apex Web Services, and Apex Unit Tests.
Question 27: What is a SOQL query, and how is it used in Apex?
SOQL (Salesforce Object Query Language) is a query language used to retrieve records from Salesforce objects. SOQL is similar to SQL but tailored for Salesforce’s data model. It allows developers to query data from standard and custom objects, apply filters, and specify which fields to retrieve.
Basic SOQL query:
apex
List<Account> accounts = [SELECT Id, Name FROM Account WHERE Industry = ‘Technology’];
In this example, the query retrieves the Id and Name fields from the Account object for all records where the Industry is set to “Technology.”
Question 28: What is SOSL, and how does it differ from SOQL?
SOSL (Salesforce Object Search Language) is used to perform full-text searches across multiple objects and fields. While SOQL is used to query specific objects, SOSL searches across multiple objects and fields, making it useful for performing global searches in Salesforce.
Basic SOSL query:
apex
List<List<SObject>> searchResults = [FIND ‘Acme’ IN ALL FIELDS RETURNING Account(Name), Contact(FirstName, LastName)];
This query searches for the term “Acme” in all fields of the Account and Contact objects and returns the Name field from Account and the FirstName and LastName fields from Contact.
Question 29: Explain the concept of sharing rules and how they affect data access in Apex.
Sharing rules in Salesforce determine the level of access users have to records. Sharing rules are used to grant additional access to records beyond what is defined by the organisation-wide defaults or role hierarchy.
In Apex, developers can enforce sharing rules by using the with sharing keyword when defining a class. This ensures that the code respects the current user’s sharing settings and only grants access to records the user is permitted to view or modify.
Example:
apex
public with sharing class MyClass {
// Code that respects sharing rules
}
If a class is defined without sharing, it bypasses sharing rules, and the code executes with full access to all records.
Question 30: What are Apex web services, and how are they used for integration?
Apex web services allow Salesforce to expose its functionality to external systems via SOAP or REST APIs. By exposing Apex methods as web services, other systems can make HTTP requests to interact with Salesforce data and execute business logic.
To define a SOAP web service in Apex, use the webService keyword:
apex
global class MyWebService {
webService static String getAccountName(Id accountId) {
Account acc = [SELECT Name FROM Account WHERE Id = :accountId];
return acc.Name;
}
}
REST web services can be defined using the @RestResource annotation:
apex
@RestResource(urlMapping=’/Account/*’)
global with sharing class AccountService {
@HttpGet
global static Account getAccountById() {
String accountId = RestContext.request.requestURI.substringAfter(‘/’);
return [SELECT Id, Name FROM Account WHERE Id = :accountId];
}
}
Apex web services are commonly used for integrating Salesforce with external systems, allowing seamless data exchange and process automation across platforms.
We’ve covered a lot of material in this article, but it’s important to remember that your interview skills are just as important as your technical knowledge. Let’s discuss some interview tips and strategies in the next section.
Salesforce Apex Interview Tips and Strategies
To increase your chances of success in your interview, let’s discuss some tips and strategies.
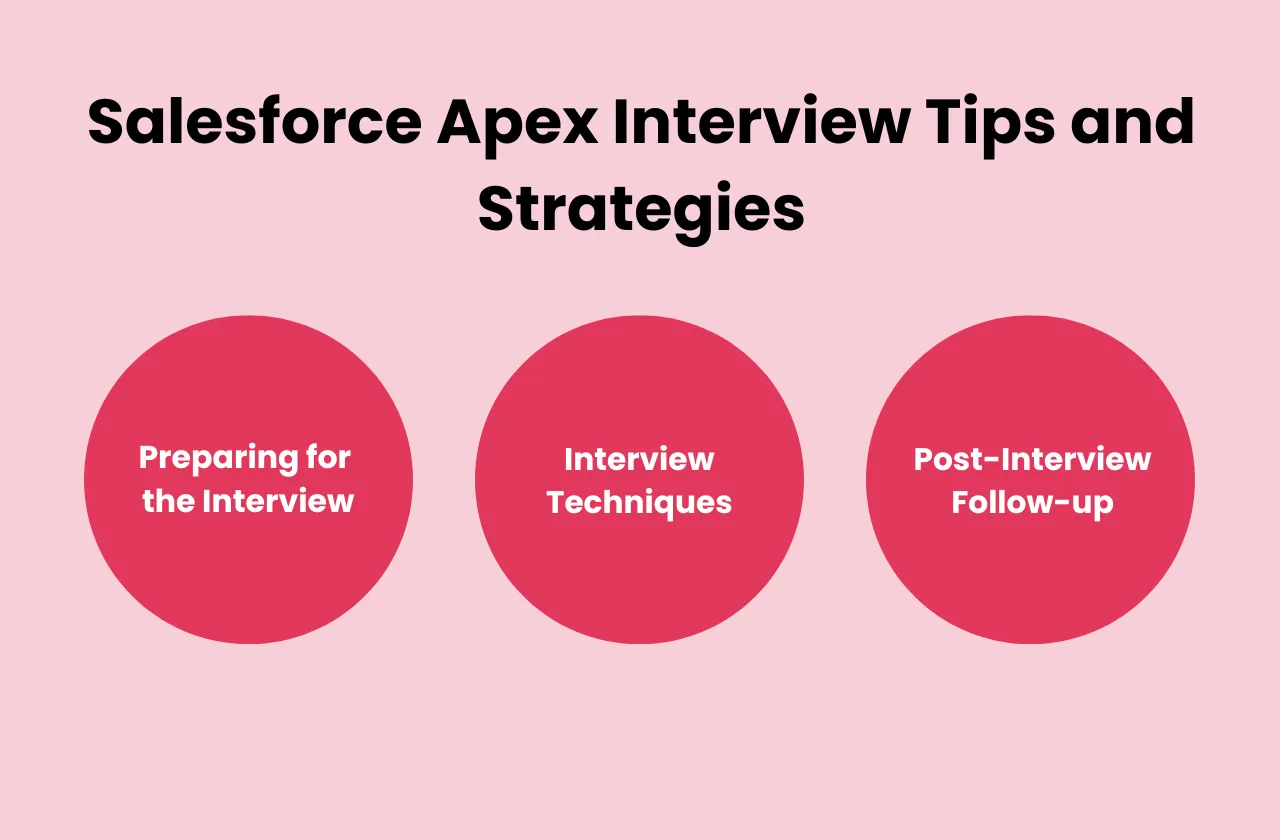
1) Preparing for the Interview
To succeed in a Salesforce Apex interview, it’s essential to thoroughly prepare:
Research common interview questions: Study frequently asked Salesforce Apex interview questions like those covered in this article. Familiarise yourself with key concepts and ensure you understand the theory behind each answer.
Practice coding exercises: Use online platforms like iScalePro to practise your coding skills. Coding challenges will help you develop a deeper understanding of Apex syntax and logic.
Understand the Salesforce platform: Ensure you have a strong understanding of how Apex fits within the broader Salesforce platform. Be prepared to answer questions about the relationships between objects, data modelling, and security settings.
2) Interview Techniques
When answering technical questions during the interview, focus on the following techniques:
Effective communication: Clearly explain your thought process as you work through coding problems. Interviewers want to understand how you approach problems, not just the final solution.
Problem-solving skills: Demonstrate your ability to solve complex problems using Salesforce Apex. If you’re unsure about a specific question, walk through the steps you would take to find a solution.
Showcase your technical skills: Use specific examples from your past projects to illustrate your experience with Apex. Mention any challenges you faced and how you overcame them.
3) Post-Interview Follow-up
After the interview, be sure to send a thank-you note to express your appreciation for the opportunity. This is a professional gesture that helps reinforce your interest in the role.
You can also follow up with any additional questions or clarifications you may have, showing your continued engagement and curiosity.
Conclusion
Mastering Salesforce Apex interview questions requires a deep understanding of Apex’s core concepts, from basic syntax to advanced topics like batch processing and web services. By studying the questions outlined in this guide, practising coding exercises, and preparing effectively for your interview, you will be well-equipped to tackle even the toughest questions with confidence.
Remember, the key to success is not just memorising answers but understanding the logic behind them and demonstrating your problem-solving abilities in a clear and concise manner.
Best of luck in your Salesforce Apex interviews!