The world of software development is always changing, and quality assurance (QA) is taking on an even bigger role. These days, automation testing is a must-have skill for testers—it helps make testing faster, more efficient, and way more reliable. One tool that stands out in this space? Selenium. It’s a powerful open-source framework that’s become essential for testing web applications. And when you pair it with Python’s flexibility and simplicity, you get a strong, easy-to-use solution for automating web browser interactions.
This article is your ultimate guide to learning Selenium with Python. By the time you’re done, you’ll have the skills and confidence to level up your automation testing game—and crush your next job interview. We’ll start with the basics, work our way through intermediate and advanced techniques, and even tackle real-world scenarios to get you ready for practical challenges. Whether you’re just getting started or you’ve been testing for a while, this guide is here to help you master Selenium with Python and navigate the exciting world of automation testing.
Python Selenium Interview Questions: Basic Questions
Basic questions test your fundamental knowledge of Selenium WebDriver. These questions cover topics like installation, configuration, and basic operations.
1) What is Selenium?
Selenium is an open-source suite of tools specifically designed for automating web browsers. It provides a single interface that lets you write test scripts in various programming languages, including Python, Java, C#, Ruby, and JavaScript. This cross-browser compatibility makes it a versatile choice for testing web applications across different environments.
Selenium is not just a single tool but a suite of tools, each catering to different testing needs:
Selenium WebDriver: This is the core of Selenium, allowing you to directly interact with web browsers using browser-specific drivers. It provides a programmatic way to control browser actions, such as navigating to pages, clicking elements, and extracting data.
Selenium IDE (Integrated Development Environment): This is a browser extension that allows you to record and playback test scripts. It’s a useful tool for beginners to get started with Selenium and for quickly creating simple test cases.
Selenium Grid: This enables parallel execution of tests across multiple machines and browsers, significantly reducing testing time and improving coverage. It’s particularly beneficial for large-scale projects and complex test suites.
2) Why use Python with Selenium?
While Selenium supports multiple programming languages, Python has emerged as a popular choice for automation testing with Selenium, and for good reason:
Ease of Use: Python is renowned for its readability and simplicity, making it an ideal language for both beginners and experienced programmers. It’s clear syntax and concise code structure reduce the learning curve and allow you to focus on the testing logic rather than getting bogged down in complex language constructs.
Extensive Library Support: Python boasts a rich ecosystem of libraries that complement Selenium for web testing. Libraries like BeautifulSoup simplify web scraping and data extraction, while libraries like pytest provide robust testing frameworks for organising and executing test cases.
Large and Active Community: Python has a vast and active community of developers and testers, providing ample resources, tutorials, and support for Selenium automation. This vibrant community ensures that you can readily find solutions to common challenges and stay updated with the latest trends.
3) How do you install Selenium in Python?
Installing Selenium in Python is a straightforward process thanks to Python’s package manager, pip. Follow these simple steps:
- Open your terminal or command prompt. This provides access to your system’s command-line interface.
- Type pip install selenium and press Enter. This command instructs pip to download and install the Selenium package from the Python Package Index (PyPI).
- Verify the installation. To confirm that Selenium is installed correctly, open a Python interpreter by typing python in your terminal. Then, type import selenium and press Enter. If no errors appear, Selenium is successfully installed.
4) What are locators in Selenium?
Locators are the fundamental building blocks of Selenium automation. They are used to identify and interact with specific elements on a web page, such as buttons, text boxes, links, and images. Choosing the right locator is crucial for creating robust and reliable tests.
Selenium supports various locator types, each with its own strengths and weaknesses:
ID: This locator uses the element’s unique id attribute. It’s generally the most reliable and efficient locator, but not all elements have an id.
Python
element = driver.find_element_by_id(“myElement”)
Name: This locator uses the element’s name attribute. It can be useful when an id is not available, but it’s less reliable as multiple elements can share the same name.
Python
element = driver.find_element_by_name(“myElement”)
XPath: This locator uses an XML path expression to navigate the HTML structure of the page and locate the element. It’s a powerful locator that can handle complex scenarios, but it can be brittle if the page structure changes.
Python
element = driver.find_element_by_xpath(“//input[@id=’myElement’]”)
CSS Selector: This locator uses CSS rules to identify the element. It’s often more concise and faster than XPath, and it’s less affected by changes in the page structure.
Python
element = driver.find_element_by_css_selector(“#myElement”)
Choosing the right locator depends on the specific element and the overall structure of the web page. Prioritise using IDs when available, and consider CSS selectors for their efficiency and maintainability.
Once you have a solid grasp of the basics, let’s move on to intermediate-level questions.
Python Selenium Interview Questions: Intermediate Questions
Intermediate questions delve deeper into Selenium WebDriver’s capabilities. These questions often involve more complex scenarios and require a strong understanding of web elements, locators, and waits.
1) What is WebDriver, and how does it work?
WebDriver is the heart of Selenium, providing a programmatic interface to control web browsers. It acts as a bridge between your test scripts and the browser, translating your commands into browser-specific actions.
Here’s how WebDriver works:
Your test script sends a command to the WebDriver API. This command could be to navigate to a URL, click a button, or enter text in a field.
The WebDriver API sends the command to a browser-specific driver. Each browser has its own driver, such as ChromeDriver for Chrome, GeckoDriver for Firefox, and IEDriverServer for Internet Explorer.
The browser driver executes the command in the browser. This results in the desired action being performed in the browser, such as opening a new page or clicking a button.
The browser driver sends the result back to the WebDriver API. This result could be the page source, the value of an element, or the status of an action.
The WebDriver API returns the result to your test script. This allows you to verify the outcome of your test and proceed with further actions.
2) How to handle dropdowns in Selenium?
Dropdowns are common elements in web forms, allowing users to select from a list of options. Selenium provides the Select class to interact with dropdown menus.
Python
from selenium.webdriver.support.ui import Select
# Find the dropdown element
dropdown = Select(driver.find_element_by_id(“myDropdown”))
# Select an option by visible text
dropdown.select_by_visible_text(“Option 1”)
# Select an option by value
dropdown.select_by_value(“option1”)
# Select an option by index
dropdown.select_by_index(2)
The Select class provides several methods to select options:
select_by_visible_text(): Selects an option based on the text displayed in the dropdown.
select_by_value(): Selects an option based on the value attribute of the option tag.
select_by_index(): Selects an option based on its index in the list (starting from 0).
3) What are implicit and explicit waits?
Waits are essential in Selenium to handle dynamic web pages that load content asynchronously. They prevent your test scripts from failing due to elements not being immediately available.
Implicit Wait: This tells WebDriver to poll the DOM for a certain amount of time when trying to find an element. If the element is not found immediately, WebDriver will keep trying for the specified duration before throwing an exception.
Python
driver.implicitly_wait(10) # Wait for up to 10 seconds
Explicit Wait: This waits for a specific condition to be met before proceeding. It provides more fine-grained control over waiting and allows you to define custom conditions.
Python
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions
as EC
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID,
“myElement”))
)
Key differences:
Scope: Implicit wait applies to all find_element calls in the driver’s lifecycle, while explicit wait applies only to the specific element and condition defined.
Flexibility: Explicit wait allows for more complex conditions and can be customised to wait for different events, while implicit wait is limited to a fixed time duration.
4) How to handle alerts and pop-ups?
Alerts and pop-ups are browser-level dialog boxes that require user interaction. Selenium provides the Alert class to handle these.
Python
# Switch to the alert
alert = driver.switch_to.alert
# Get the alert text
alert_text = alert.text
# Accept the alert (click OK)
alert.accept()
# Dismiss the alert (click Cancel)
alert.dismiss()
# Enter text in the alert (if it has an input field)
alert.send_keys(“some text”)
The Alert class provides methods to:
accept(): Click the “OK” button.
dismiss(): Click the “Cancel” button.
send_keys(): Enter text into the alert box.
5) Explain the difference between find_element and find_elements.
Both methods are used to locate elements on a web page, but they differ in their return types and how they handle situations where no matching element is found:
find_element: This method returns the first element that matches the given locator. If no matching element is found, it throws a NoSuchElementException.
Python
element = driver.find_element_by_id(“myElement”)
find_elements: This method returns a list of all elements that match the given locator. If no matching element is found, it returns an empty list.
Python
elements = driver.find_elements_by_class_name(“myClass”)
Use find_element when you expect only one matching element and want to interact with it directly. Use find_elements when you need to work with multiple elements or want to check the presence or absence of elements.
To prepare for more challenging roles, you’ll need to be comfortable with advanced Selenium concepts. Let’s explore some advanced interview questions.
Python Selenium Interview Questions: Advanced Questions
Advanced questions assess your expertise in Selenium WebDriver and your ability to handle complex automation tasks. These questions often involve topics like page object model, test frameworks, and parallel testing.
1) How to handle multiple browser tabs?
Modern web applications often involve interactions with multiple browser tabs. Selenium provides the window_handles property to manage these tabs.
Python
# Get the current window handle
main_window = driver.current_window_handle
# Open a new tab (e.g., by clicking a link)
# Get all window handles
all_windows = driver.window_handles
# Switch to the new tab
for window in all_windows:
if window != main_window:
driver.switch_to.window(window)
break
# Perform actions in the new tab
# Switch back to the main tab
driver.switch_to.window(main_window)
The window_handles property returns a list of window handles, each representing an open tab. You can use the switch_to.window() method to switch between tabs by providing the desired window handle.
2) Explain how to execute JavaScript using Selenium.
Selenium allows you to execute JavaScript code within the browser context using the execute_script method. This can be useful for tasks that are not directly supported by Selenium’s API, such as scrolling, manipulating the DOM, or triggering events.
Python
# Scroll to the bottom of the page
driver.execute_script(“window.scrollTo(0, document.body.scrollHeight);”)
# Get the value of an attribute
attribute_value = driver.execute_script(
“return document.getElementById(‘myElement’).getAttribute(‘value’);”
)
# Trigger a click event
driver.execute_script(“arguments[0].click();”, element)
The execute_script method takes a JavaScript code snippet as its first argument. You can also pass arguments to your JavaScript code using arguments[0], arguments[1], etc.
3) How do you upload a file using Selenium?
File uploads are common in web applications, and Selenium provides ways to handle them. The most common approach is to use the send_keys method on the file input element, providing the path to the file you want to upload.
Python
# Find the file upload element
upload_element = driver.find_element_by_id(“fileUpload”)
# Enter the file path
upload_element.send_keys(“/path/to/your/file.txt”)
In some cases, you might encounter a browser-level file upload dialog box. Handling these dialogs can be tricky as they are outside the scope of Selenium’s control. You might need to use AutoIT or other tools to automate these interactions.
4) What are some common exceptions in Selenium?
Selenium WebDriver can throw various exceptions during test execution, indicating errors or unexpected conditions. Here are some common exceptions:
TimeoutException: This occurs when an operation takes longer than the specified time limit, such as waiting for an element to appear or a page to load.
NoSuchElementException: This occurs when WebDriver cannot find an element with the given locator. This could be due to an incorrect locator, the element not being present on the page, or the element not being loaded yet.
StaleElementReferenceException: This occurs when the element you are trying to interact with is no longer attached to the DOM. This can happen if the page has been refreshed or the element has been removed dynamically.
ElementNotVisibleException: This occurs when the element is present in the DOM but is not visible on the page. This could be because the element is hidden by CSS or is located outside the viewport.
ElementNotInteractableException: This occurs when the element is present and visible but cannot be interacted with, such as clicking a disabled button or entering text in a read-only field.
Understanding these exceptions and their causes is crucial for debugging your test scripts and creating robust tests. Use try-except blocks to handle exceptions gracefully and provide informative error messages.
In addition to technical questions, you may also be asked scenario-based questions to evaluate your problem-solving and automation skills.
Python Selenium Interview Questions: Scenario-Based Questions
Scenario-based questions simulate real-world automation challenges. These questions require you to apply your knowledge of Selenium WebDriver to solve specific problems.
1) Write a script to scrape data from a website.
Web scraping involves extracting data from websites. Selenium, combined with a library like BeautifulSoup, can be used for this task.
Python
from selenium import webdriver
from bs4 import BeautifulSoup
# Set up the WebDriver
driver = webdriver.Chrome()
# Go to the website
driver.get(“https://www.example.com”)
# Get the page source
page_source = driver.page_source
# Parse the page source with BeautifulSoup
soup = BeautifulSoup(page_source, “html.parser”)
# Find
the elements you want to scrape
titles = soup.find_all(“h2″, class_=”title”)
# Extract the data
for title in titles:
print(title.text)
# Close the browser
driver.quit()
This script uses Selenium to navigate to a website and retrieve the page source. BeautifulSoup then parses the HTML and extracts the text content of all h2 elements with the class “title”.
2) How to test login functionality using Selenium?
Testing login functionality is a common scenario in web application testing. Here’s how you can automate this using Selenium:
Python
# Go to the login page
driver.get(“https://www.example.com/login”)
# Find the username and password fields
username_field = driver.find_element_by_id(“username”)
password_field = driver.find_element_by_id(“password”)
# Enter the username and password
username_field.send_keys(“your_username”)
password_field.send_keys(“your_password”)
# Click the login button
login_button = driver.find_element_by_id(“login_button”)
login_button.click()
# Check if the login was successful (e.g., by looking for a welcome message)
welcome_message = driver.find_element_by_id(“welcome_message”)
assert welcome_message.text == “Welcome, your_username!”
This script navigates to the login page, enters the username and password, clicks the login button, and then asserts that a welcome message is displayed, indicating successful login.
3) Automate a test case to perform form submission.
Form submission is another common scenario in web testing. Here’s how you can automate it:
Python
# Go to the form page
driver.get(“https://www.example.com/form”)
# Fill in the form fields
name_field = driver.find_element_by_id(“name”)
name_field.send_keys(“John Doe”)
email_field = driver.find_element_by_id(“email”)
email_field.send_keys(“john.doe@example.com”)
# Handle a dropdown
dropdown = Select(driver.find_element_by_id(“country”))
dropdown.select_by_visible_text(“United States”)
# Handle a checkbox
checkbox = driver.find_element_by_id(“agree”)
checkbox.click()
# Submit the form
submit_button = driver.find_element_by_id(“submit”)
submit_button.click()
# Check if the form submission was successful (e.g., by looking for a confirmation message)
confirmation_message = driver.find_element_by_id(“confirmation”)
assert confirmation_message.text ==
“Form submitted successfully!”
This script fills in various form fields, including text boxes, a dropdown, and a checkbox, and then submits the form. It then checks for a confirmation message to verify successful submission.
4) Validate if a web element is displayed and enabled.
Before interacting with a web element, you might need to check if it’s visible and enabled. Selenium provides methods for this:
Python
# Find the element
element = driver.find_element_by_id(“myElement”)
# Check if it is displayed
if element.is_displayed():
print(“Element is displayed.”)
# Check if it is enabled
if element.is_enabled():
print(“Element is enabled.”)
The is_displayed() method checks if the element is visible on the page, while is_enabled() checks if the element is interactable, such as a button that is not disabled.
To increase your chances of success in a Python Selenium interview, it’s essential to follow best practices.
Python Selenium Interview Best Practices
Beyond answering specific questions, interviewers often assess your understanding of best practices in Selenium automation. Here are some key areas to focus on:
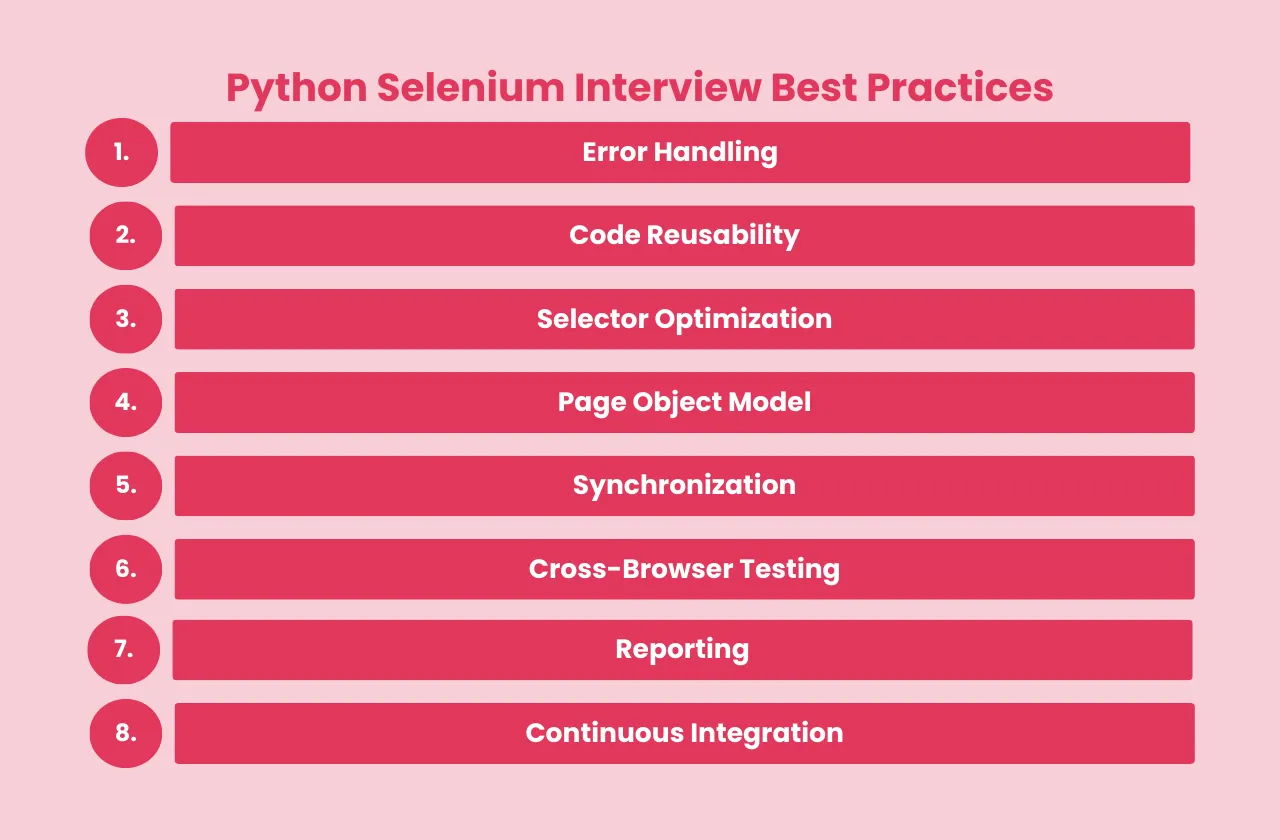
Error Handling: Robust test scripts should anticipate and handle potential errors gracefully. Use try-except blocks to catch exceptions like TimeoutException, NoSuchElementException, and StaleElementReferenceException. This prevents your tests from abruptly failing and provides informative error messages for debugging.
Code Reusability: Create reusable functions and classes to avoid code duplication and improve maintainability. This promotes modularity and makes your test scripts easier to understand and update.
Selector Optimization: Choose efficient locators, prioritising IDs and CSS selectors over XPath when possible. Well-crafted locators improve test execution speed and reduce the likelihood of tests breaking due to changes in the page structure.
Page Object Model (POM): Consider using the POM design pattern to organise your test code and separate page-specific logic from test scripts. POM improves code readability, maintainability, and reusability.
Synchronisation: Handle dynamic elements and asynchronous actions using appropriate waits, such as implicit and explicit waits. This ensures that your tests interact with elements only when they are available and in the expected state.
Cross-Browser Testing: Test your web application across different browsers and platforms to ensure compatibility and consistent behaviour. Selenium Grid facilitates parallel execution on multiple browsers, saving time and effort.
Reporting: Generate clear and concise test reports to track test results, identify failures, and analyse trends. Frameworks like pytest provide built-in reporting capabilities, or you can use dedicated reporting libraries.
Continuous Integration: Integrate your Selenium tests with a continuous integration (CI) system like Jenkins or Travis CI to automate test execution as part of your development workflow.
To further enhance your Selenium skills, let’s explore some advanced concepts and techniques.
Delving Deeper into Selenium WebDriver: Advanced Concepts and Techniques
Beyond the basics, there are advanced Selenium concepts and techniques that can elevate your automation skills. These include topics like headless browser testing, mobile automation, and API testing with Selenium.
1) Interacting with Web Elements:
While basic interactions like clicking and typing are straightforward, Selenium offers a richer set of actions for handling various web elements:
Submitting Forms: Instead of clicking a submit button, you can directly submit a form using submit():
Python
form = driver.find_element_by_id(“myForm”)
form.submit()
Extracting Element Attributes: Get the value of an element’s attribute using get_attribute():
Python
link = driver.find_element_by_tag_name(“a”)
href = link.get_attribute(“href”)
Working with Checkboxes and Radio Buttons: Interact with these elements using is_selected() and click():
Python
checkbox = driver.find_element_by_id(“myCheckbox”)
if not checkbox.is_selected():
checkbox.click()
Handling Drag and Drop: Simulate drag-and-drop actions using the ActionChains class:
Python
source = driver.find_element_by_id(“draggable”)
target = driver.find_element_by_id(“droppable”)
ActionChains(driver).drag_and_drop(source, target).perform()
2) Navigating Web Pages:
Go beyond simple navigation with these techniques:
Navigating Back and Forward: Use back() and forward() to simulate browser history navigation:
Python
driver.get(“https://www.example.com/page1”)
driver.get(“https://www.example.com/page2”)
driver.back() # Go back to page1
driver.forward() # Go forward to page2
Refreshing the Page: Refresh the current page using refresh():
Python
driver.refresh()
Working with Frames: Switch to frames using switch_to.frame():
Python
driver.switch_to.frame(“myFrame”)
# Interact with elements within the frame
driver.switch_to.default_content() # Switch back to the main content
3) Handling Dynamic Content:
Modern web applications often load content dynamically using AJAX or JavaScript. Selenium provides ways to handle this:
Explicit Waits with Expected Conditions: Wait for specific conditions like element visibility, presence, or clickability:
Python
from selenium.webdriver.support import expected_conditions as EC
element = WebDriverWait(driver, 10).until(
EC.element_to_be_clickable((By.ID,
“myElement”))
)
Fluent Wait: Define custom polling intervals and ignore specific exceptions while waiting:
Python
from selenium.webdriver.support.ui import FluentWait
wait = FluentWait(driver) \
.with_timeout(10, TimeUnit.SECONDS) \
.polling_every(1, TimeUnit.SECONDS) \
.ignoring(NoSuchElementException)
element = wait.until(lambda driver: driver.find_element_by_id(“myElement”))
4) Advanced Locators:
Mastering advanced locator techniques can be invaluable for handling complex scenarios:
XPath Axes: Navigate the DOM tree using axes like parent, child, following-sibling, and preceding-sibling:
Python
# Find the parent of an element
parent = element.find_element_by_xpath(“./parent::*”)
# Find the following sibling of an element
sibling = element.find_element_by_xpath(“./following-sibling::div”)
CSS Selector Combinators: Combine selectors using combinators like >, +, and :
Python
# Find a direct child of an element
child = driver.find_element_by_css_selector(“div > p”)
# Find an element immediately following another element
following = driver.find_element_by_css_selector(“h2 + p”)
5) Taking Screenshots and Logging:
Capture screenshots for debugging and reporting:
Python
driver.save_screenshot(“screenshot.png”)
Implement logging to track test execution and identify issues:
Python
import logging
logging.basicConfig(filename=”test.log”, level=logging.INFO)
logging.info(“Test started”)
# … test actions …
logging.info(“Test completed”)
By mastering these advanced concepts, you can become a more versatile and valuable automation engineer.
Beyond the Basics: Enhancing Your Selenium Skillset
To stay ahead in the field of automation testing, continuous learning is essential. Explore additional tools and frameworks that complement Selenium, such as TestNG, JUnit, and Appium.
1) Headless Browser Testing:
Run Selenium tests without a visible browser window using headless browsers like Chrome and Firefox. This is beneficial for CI/CD environments and performance testing.
Python
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_argument(“–headless”)
driver = webdriver.Chrome(options=chrome_options)
2) Handling Cookies:
Manage browser cookies for testing scenarios that involve user sessions and authentication.
Python
# Get all cookies
cookies = driver.get_cookies()
# Add a cookie
driver.add_cookie({“name”: “myCookie”, “value”: “myValue”})
# Delete a cookie
driver.delete_cookie(“myCookie”)
# Delete all cookies
driver.delete_all_cookies()
3) Network Interception and Mocking:
Use tools like BrowserMob Proxy to intercept network requests and responses, allowing you to simulate network conditions, modify headers, and mock API responses.
4) Performance Testing with Selenium:
While Selenium is primarily for functional testing, you can use it in conjunction with tools like JMeter or LoadRunner to measure web application performance.
5) Mobile Testing with Appium:
Appium extends Selenium to automate mobile app testing on iOS and Android platforms. It uses the WebDriver protocol to interact with mobile apps, allowing you to reuse your Selenium skills for mobile automation.
By combining your knowledge of Python Selenium with these advanced concepts and techniques, you can excel in your automation career.
Conclusion
This comprehensive guide has equipped you with the knowledge and tools to excel in your Python Selenium interviews and your automation testing journey. By mastering the concepts, techniques, and best practices outlined here, you’ll be well-prepared to tackle real-world testing challenges and contribute effectively to software quality assurance.
Remember that continuous learning and practice are key to staying ahead in the ever-evolving field of automation testing. Explore new tools, libraries, and frameworks, and keep refining your skills to become a proficient and sought-after automation tester.