Infosys is one of the leading IT companies in India and is known for its rigorous selection process. Two of the most sought-after roles at Infosys are the Specialist Programmer (SP) and Digital Specialist Engineer (DSE) positions. These roles are highly competitive, and the coding round is a crucial part of the selection process. For job seekers aiming to secure a position as an Infosys SP or DSE, mastering the coding round is essential.
Coding skills are vital in the selection process for these roles because they demonstrate a candidate’s problem-solving abilities and technical knowledge. This article provides a comprehensive guide to understanding the Infosys SP and DSE coding round, common coding questions, tips for improving coding skills, and how to avoid common coding mistakes.
Understanding the Infosys SP And DSE Coding Round
The coding round is a critical component of the Infosys SP and DSE selection process. Understanding the format, difficulty levels, and common topics can help candidates prepare effectively.
Format of the Infosys SP And DSE Coding Round
In the Infosys SP and DSE coding round, candidates are typically given 2-3 coding questions to solve within a set time limit, usually around 60-120 minutes. The languages allowed for coding can vary, but candidates often have the option to choose from popular programming languages such as Python, Java, C++, and C.
The coding round is designed to test a candidate’s ability to write efficient and accurate code. The questions range in difficulty, and candidates must manage their time effectively to complete all the questions within the allotted time.
Difficulty Levels of Questions
The coding round includes questions of varying difficulty levels:
Easy: These questions are straightforward and test basic programming concepts. They usually require knowledge of basic data structures, algorithms, and simple problem-solving techniques.
Medium: Medium-level questions are more complex and may involve multiple steps to solve. They often require a good understanding of algorithms and data structures.
Hard: Hard questions are the most challenging and require advanced problem-solving skills. These questions often involve dynamic programming, complex graph algorithms, and other advanced topics.
Common Topics Covered in the Infosys SP And DSE Coding Round
Candidates should focus on the following topics when preparing for the Infosys SP and DSE coding round:
Data Structures: Arrays, linked lists, stacks, and queues are commonly tested in the coding round. Understanding how to implement and manipulate these data structures is essential.
Algorithms: Sorting and searching algorithms, greedy algorithms, backtracking, and dynamic programming are key topics. Candidates should practise implementing these algorithms to solve complex problems.
Problem-Solving: Problem-solving skills are crucial in the coding round. Candidates should practise solving problems that require logical thinking and the application of algorithms.
With a clear grasp of the coding round format, candidates can focus on the types of questions they will face. Next, we will look at some common coding questions asked in these interviews.
Infosys SP And DSE Coding Questions and Answers
To succeed in the Infosys SP and DSE coding round, candidates should familiarise themselves with common coding questions. Below are examples of questions at different difficulty levels, along with explanations and code snippets.
Infosys SP And DSE Easy Level Coding Questions
Basic Data Structures
Example: Given an array of integers, find the second largest element.
Explanation: To solve this problem, iterate through the array to find the largest element, then iterate again to find the second largest element.
Code Snippet (Python):
python
def second_largest(arr):
first, second = float(‘-inf’), float(‘-inf’)
for num in arr:
if num > first:
second = first
first = num
elif first > num > second:
second = num
return second
String Manipulation
Example: Reverse a given string without using any built-in functions.
Explanation: Use a loop to swap characters from the beginning and end of the string until you reach the middle.
Code Snippet (Python):
python
def reverse_string(s):
n = len(s)
for i in range(n // 2):
s[i], s[n – i – 1] = s[n – i – 1], s[i]
return ”.join(s)
Searching and Sorting Algorithms
Example: Implement a binary search algorithm.
Explanation: Binary search works by repeatedly dividing the search interval in half. Start with the entire array, then check the middle element. If the target is smaller, narrow the search to the lower half; if larger, narrow it to the upper half.
Code Snippet (Python):
python
def binary_search(arr, target):
low, high = 0, len(arr) – 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid – 1
return -1
Mathematical Problems
Example: Find the greatest common divisor (GCD) of two numbers.
Explanation: The Euclidean algorithm is an efficient method for computing the GCD of two numbers.
Code Snippet (Python):
python
def gcd(a, b):
while b:
a, b = b, a % b
return a
Infosys SP And DSE Medium Level Coding Questions
Greedy Algorithms
Example: Given an array of coins, find the minimum number of coins needed to make a specific amount.
Explanation: Use a greedy algorithm to select the largest possible denomination first, then move to the next largest, and so on.
Code Snippet (Python):
python
def min_coins(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
count += amount // coin
amount %= coin
return count if amount == 0 else -1
Backtracking
Example: Solve the N-Queens problem.
Explanation: Use backtracking to place queens on a chessboard such that no two queens threaten each other.
Code Snippet (Python):
python
def solve_n_queens(n):
def is_safe(board, row, col):
for i in range(col):
if board[row][i] == 1:
return False
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0
return False
board = [[0] * n for _ in range(n)]
if not solve(board, 0):
return []
return board
Dynamic Programming (Basic Concepts)
Example: Find the longest increasing subsequence in an array.
Explanation: Use dynamic programming to store the length of the longest increasing subsequence ending at each element.
Code Snippet (Python):
python
def longest_increasing_subsequence(arr):
dp = [1] * len(arr)
for i in range(1, len(arr)):
for j in range(i):
if arr[i] > arr[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
Graph Algorithms (BFS, DFS)
Example: Find the shortest path in an unweighted graph using BFS.
Explanation: Use the Breadth-First Search (BFS) algorithm to explore the graph level by level and find the shortest path.
Code Snippet (Python):
python
from collections import deque
def bfs_shortest_path(graph, start, goal):
visited = set()
queue = deque([[start]])
if start == goal:
return [start]
while queue:
path = queue.popleft()
node = path[-1]
if node not in visited:
neighbors = graph[node]
for neighbor in neighbors:
new_path = list(path)
new_path.append(neighbor)
queue.append(new_path)
if neighbor == goal:
return new_path
visited.add(node)
return []
Infosys SP And DSE Hard Level Coding Questions
Advanced Dynamic Programming
Example: Solve the knapsack problem using dynamic programming.
Explanation: Use a dynamic programming table to store the maximum value that can be obtained for each weight limit.
Code Snippet (Python):
python
def knapsack(weights, values, capacity):
n = len(values)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(dp[i-1][w], dp[i-1][w-weights[i-1]] + values[i-1])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
Graph Algorithms (Dijkstra, Floyd-Warshall)
Example: Implement Dijkstra’s algorithm to find the shortest path in a weighted graph.
Explanation: Dijkstra’s algorithm finds the shortest paths from a source vertex to all other vertices in a graph with non-negative edge weights.
Code Snippet (Python):
python
import heapq
def dijkstra(graph, start):
heap = [(0, start)]
distances = {vertex: float(‘inf’) for vertex in graph}
distances[start] = 0
while heap:
current_distance, current_vertex = heapq.heappop(heap)
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex]:
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(heap, (distance, neighbor))
return distances
Combinatorics and Probability
Example: Calculate the number of unique paths from the top-left to the bottom-right corner of a grid.
Explanation: This problem can be solved using combinatorial mathematics, specifically the binomial coefficient.
Code Snippet (Python):
python
def unique_paths(m, n):
def factorial(x):
if x == 0 or x == 1:
return 1
return x * factorial(x – 1)
return factorial(m + n – 2) // (factorial(m – 1) * factorial(n – 1))
Geometric Algorithms
Example: Determine if three given points form a triangle and calculate its area if they do.
Explanation: Use the determinant of a matrix formed by the points to determine if they are collinear. If not, apply the shoelace formula to compute the area.
Code Snippet (Python):
python
def is_triangle(p1, p2, p3):
return not (p1[0] * (p2[1] – p3[1]) + p2[0] * (p3[1] – p1[1]) + p3[0] * (p1[1] – p2[1]) == 0)
def triangle_area(p1, p2, p3):
return abs(p1[0] * (p2[1] – p3[1]) + p2[0] * (p3[1] – p1[1]) + p3[0] * (p1[1] – p2[1])) / 2
By familiarising themselves with these common questions, candidates can better prepare for the coding round. Now, let’s discuss some effective tips for improving coding skills specifically for the Infosys coding round.
Tips for Improving Infosys SP And DSE Coding Skills
Success in coding rounds for Infosys SP and DSE roles demands not just knowledge but also skill development. Here are some tips to enhance your coding skills.
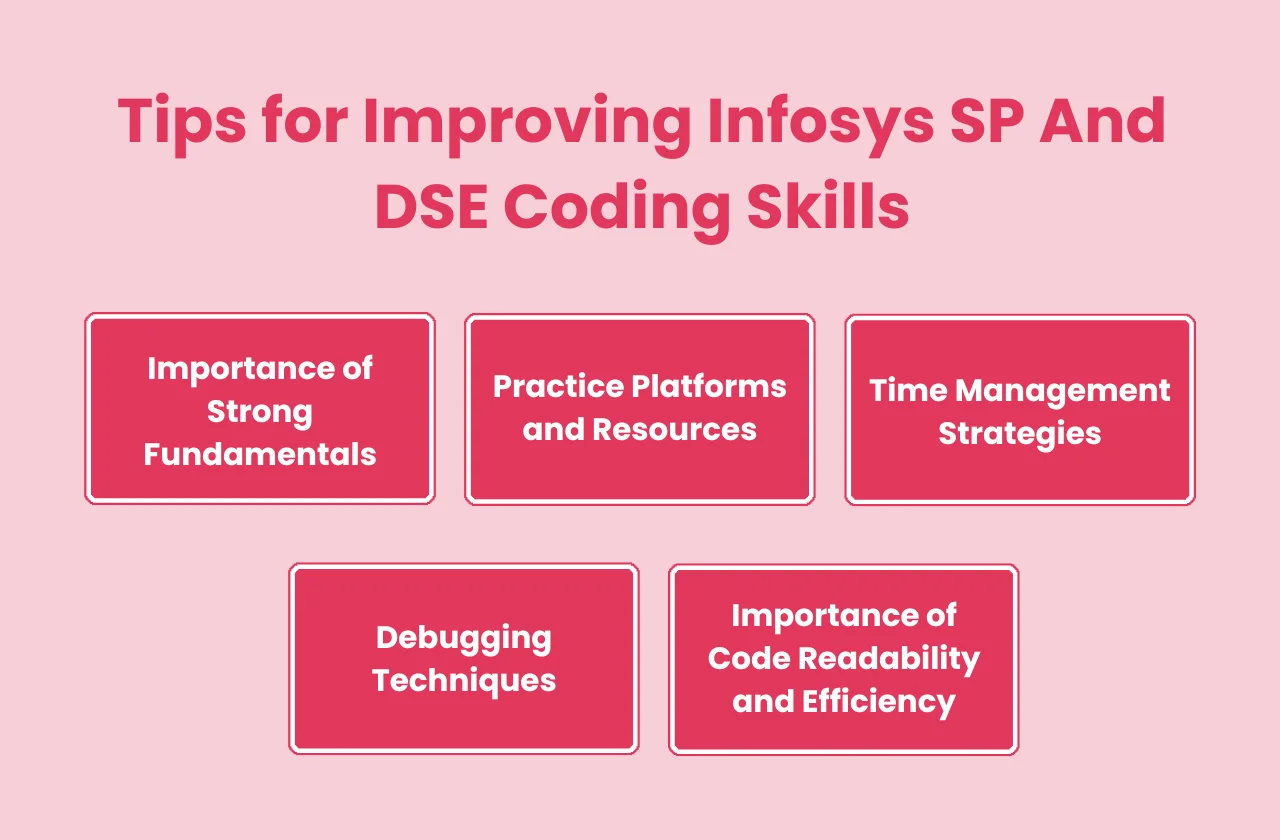
1) Importance of Strong Fundamentals
Before diving into advanced topics, ensure you have a solid grasp of the basics. Concepts like loops, arrays, strings, recursion, and fundamental data structures (like stacks, queues, and linked lists) form the foundation upon which more complex knowledge is built. Regularly revisiting these basics and practising related problems can strengthen your problem-solving abilities.
2) Practice Platforms and Resources
Practice is crucial to mastering coding. Use online platforms like LeetCode, HackerRank, Codeforces, and iScalePro to solve a wide range of problems. These platforms offer problems categorised by difficulty level, which is beneficial for structured learning. Practising on these platforms not only enhances your coding skills but also familiarises you with the type of questions that might appear in the coding round.
3) Time Management Strategies
Time management is key during coding rounds. Start by solving easier problems to build momentum, then gradually tackle more difficult ones. Keep track of time as you solve each problem. Practice under timed conditions to simulate the pressure of a real exam, and work on improving your speed without compromising accuracy.
4) Debugging Techniques
Debugging is an essential skill in coding. Learn to read error messages and understand the underlying issues in your code. Use print statements or debugging tools to trace the flow of your program and identify where things go wrong. Developing a systematic approach to debugging will help you fix errors quickly and efficiently during the coding round.
5) Importance of Code Readability and Efficiency
Writing clean, readable code is just as important as writing correct code. Use meaningful variable names, write comments to explain complex sections, and maintain a consistent coding style. Additionally, focus on code efficiency—optimise your algorithms to reduce time and space complexity. This not only ensures that your code runs faster but also demonstrates your attention to detail and understanding of best practices.
Implementing these strategies can significantly improve a candidate’s coding abilities. Next, we will address common coding mistakes and how to avoid them during the Infosys interview.
Common Coding Mistakes and How to Avoid Them for Infosys SP And DSE Interview
Even well-prepared candidates can make mistakes during the coding round. Being aware of common errors and knowing how to avoid them can make a big difference.
1) Common Errors Made by Candidates
Not Reading the Problem Statement Carefully: Many candidates rush through the problem statement, missing critical details. This leads to incorrect assumptions and ultimately, incorrect solutions.
Poor Time Management: Spending too much time on a single problem without making progress can prevent you from completing other questions that you could have solved.
Overcomplicating Solutions: Some candidates try to use advanced algorithms when a simple approach would suffice. This can lead to unnecessary complexity and errors.
Ignoring Edge Cases: Failing to consider edge cases, such as empty inputs or maximum limits, can result in your solution failing in unexpected scenarios.
Not Testing Thoroughly: Candidates sometimes submit code without thoroughly testing it with various inputs. This can lead to incomplete solutions that fail in some cases.
2) How to Identify and Rectify These Errors
Carefully Read the Problem Statement: Take the time to thoroughly read and understand the problem statement. Identify the inputs, outputs, and any constraints before starting your solution.
Plan Your Approach: Before jumping into coding, plan your approach. Break down the problem into smaller parts, and think about the most efficient way to solve each part.
Use Simple Solutions When Possible: If a problem can be solved with a simple solution, go with it. Avoid overcomplicating your code unnecessarily.
Consider Edge Cases: Always think about edge cases and test your code against them. Consider the smallest, largest, and most unusual inputs your code might encounter.
Test Thoroughly: Test your code with a variety of inputs, including normal cases, edge cases, and even invalid inputs (if applicable). This ensures your solution is robust and reliable.
3) Best Practices for Writing Clean and Efficient Code
Use Meaningful Names: Use clear and descriptive names for your variables, functions, and classes. This makes your code easier to understand and maintain.
Write Modular Code: Break your code into smaller functions or modules that each handle a specific task. This not only makes your code more organised but also makes it easier to test and debug.
Avoid Hard-Coding Values: Avoid using hard-coded values in your code. Instead, use variables or constants that can be easily modified if needed.
Optimise for Efficiency: Always consider the time and space complexity of your solutions. Look for ways to make your code run faster and use less memory.
Keep Code Consistent: Maintain a consistent coding style throughout your solution. This includes consistent indentation, naming conventions, and commenting.
Conclusion
Preparing for the Infosys SP and DSE coding round requires a combination of strong fundamentals, regular practice, and attention to detail. By understanding the format and difficulty levels of the coding round, familiarising yourself with common coding questions, and following best practices, you can significantly improve your chances of success.
Remember to practise on platforms like iScalePro and use the tips provided in this article to enhance your coding skills. Avoid common mistakes by reading problem statements carefully, managing your time effectively, and thoroughly testing your solutions. Writing clean, efficient, and readable code is crucial in demonstrating your technical abilities during the coding round.
With dedication and the right preparation, you can excel in the coding round and move one step closer to securing a coveted role as an Infosys Specialist Programmer or Digital Specialist Engineer.