Job seekers often find coding interviews difficult. They struggle to find up-to-date questions and clear answers. This lack of preparation can lead to missed job opportunities.
This article understands your concern. It provides the latest Accenture coding questions along with detailed answers. We hope it helps you prepare well and land your dream job at Accenture.
Understanding Accenture’s Coding Assessment
Accenture’s coding assessment is a key part of their hiring process. It tests your coding skills and how well you solve problems. Here’s what you need to know:
Common Question Types
You’ll face different types of questions to show your skills:
- Data Structures and Algorithms: These are the building blocks of coding. Expect questions on:
- Array manipulation: Changing and working with lists of data.
- String manipulation: Working with text, like finding specific words or changing their order.
- Linked lists, trees, graphs: Different ways to organise data with connections.
- Sorting: Putting data in order.
- Searching: Finding specific data in a set.
- Dynamic programming: Solving complex problems by breaking them into smaller, easier steps.
- Problem-Solving: These questions test your logical thinking. You might get:
- Logic puzzles: Questions that make you think step-by-step.
- Mathematical problems: Using maths to find answers.
- Coding Challenges: You might need to write code to:
- Implement functions: Small pieces of code that do specific tasks.
- Create classes: Blueprints for making objects in your code.
Programming Languages
Accenture usually lets you choose a programming language you know well. Common choices are:
- Java: A popular language used for many different kinds of applications.
- Python: A beginner-friendly language known for being easy to read.
- C++: A powerful language often used for system-level programming.
Time Constraints
You’ll have a limited time to answer questions. This tests how fast you can think and code. Usually, you get:
- 45 minutes: For two coding questions.
- 15 minutes: For multiple-choice questions on algorithms.
Here’s how to manage your time:
- Read Carefully: Make sure you understand the question before starting.
- Plan: Think about how you’ll solve the problem before writing code.
- Code: Write clear and simple code. Don’t worry about making it perfect at first.
- Test: Run your code to check for mistakes.
- Improve: If you have time, make your code better.
Accenture Coding Concepts for the Interview
Accenture coding interviews test your understanding of fundamental programming concepts. Let’s break down the key areas you should master:
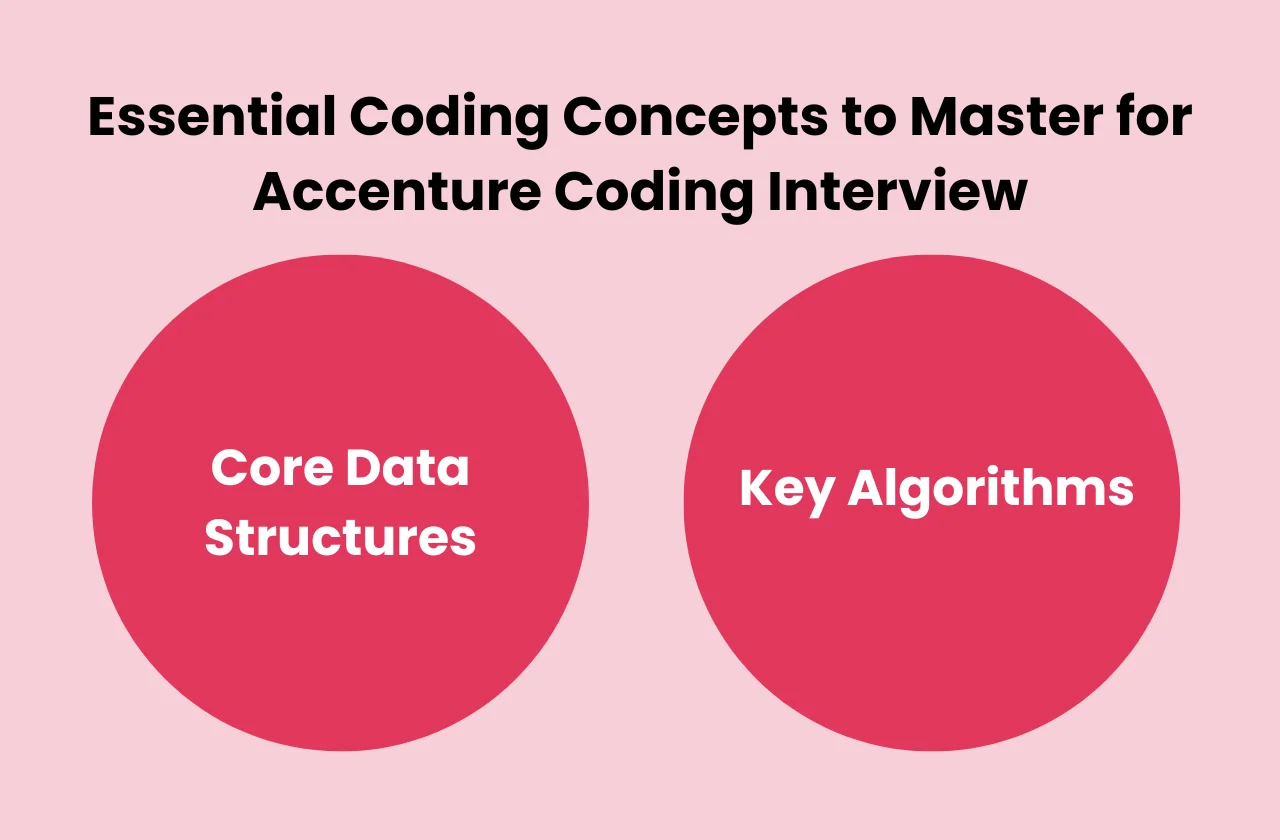
Core Data Structures
Data structures are the building blocks for organising and storing information in your programs.
- Arrays and Lists:
- Arrays hold items of the same type in a continuous block of memory.
- Lists can hold items of different types and may not be stored contiguously.
- Use arrays when you know the size beforehand and need fast access by index. Use lists when you need flexibility to add or remove items easily.
- Linked Lists:
- Linked lists are chains of nodes, where each node stores data and a link to the next node.
- Use linked lists when you need to insert or delete items frequently within the list, as these operations are often faster than in arrays.
- Stacks and Queues:
- Stacks follow a “last in, first out” (LIFO) order. Imagine a stack of plates.
- Queues follow a “first in, first out” (FIFO) order. Think of a line of people.
- Trees:
- Trees are hierarchical structures with a root node and branches connecting parent nodes to child nodes.
- Binary trees have at most two children per node.
- Binary search trees are a special type of binary tree where the left child is smaller and the right child is larger than the parent.
- Graphs:
- Graphs represent relationships between objects. They consist of nodes (vertices) connected by edges.
- Social networks, road maps, and airline routes are examples of systems that can be modelled as graphs.
- Hash Tables:
- Hash tables use a hash function to map keys to values. They offer fast lookups, insertions, and deletions.
- Use hash tables when you need quick access to data based on a key.
Key Algorithms
Algorithms are sets of instructions for solving specific problems.
- Sorting:
- Sorting algorithms arrange data in a specific order (ascending, descending, etc.).
- Bubble Sort is simple but slow.
- Quick Sort and Merge Sort are generally faster for larger datasets.
- Searching:
- Searching algorithms find specific items within a collection of data.
- Linear search checks items one by one.
- Binary search is faster for sorted data, working by repeatedly dividing the search interval in half.
- Recursion:
- Recursion is a technique where a function calls itself to solve smaller subproblems.
- Factorial calculation and tree traversal are common examples of recursion.
- Dynamic Programming:
- Dynamic programming solves complex problems by breaking them down into simpler overlapping subproblems.
- It stores the results of subproblems to avoid redundant calculations.
- The Fibonacci sequence is a classic example solved with dynamic programming.
- String Manipulation Algorithms:
- These algorithms work with text data.
- Examples include finding palindromes, reversing strings, or checking if strings are anagrams.
- Graph Traversal Algorithms:
- Breadth-First Search (BFS) explores all the neighbour nodes at the present depth before moving on to nodes at the next depth level.
- Depth-First Search (DFS) explores as far as possible along each branch before backtracking.
Accenture Coding Questions & Answers
Accenture is a leading global professional services company that is known for its rigorous coding interviews. To excel in the Accenture coding round, it is essential to practise a wide range of coding questions that cover different levels of difficulty.Â
In this article, we will explore several Accenture coding questions, categorised into beginner, intermediate, and advanced levels, along with detailed solutions and analysis.
Accenture Coding Questions: Beginner-Level
Array Reversal
Problem Statement:
Given an array of integers, write a function to reverse the order of the elements in the array.
Approach:
One simple approach to reversing an array is to use two pointers – one at the beginning and one at the end of the array. Swap the elements at these two pointers, then move the pointers inward until they meet in the middle.
Solution:
python
def reverse_array(arr):
left = 0
right = len(arr) – 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
Time Complexity: O(n), where n is the length of the input array.
Space Complexity: O(1), as we are modifying the input array in-place.
Finding Duplicates in an Array
Problem Statement:
Given an array of integers, write a function to find all the duplicate elements in the array.
Approach:
One approach is to use a hash table (dictionary in Python, HashMap in Java) to keep track of the frequency of each element in the array. Then, we can iterate through the hash table and add the elements with a frequency greater than 1 to the result list.
Solution:
python
def find_duplicates(arr):
freq = {}
for num in arr:
if num in freq:
freq[num] += 1
else:
freq[num] = 1
duplicates = [num for num, count in freq.items() if count > 1]
return duplicates
Time Complexity: O(n), where n is the length of the input array.
Space Complexity: O(n), as we are using a hash table to store the frequency of each element.
Fibonacci Sequence
Problem Statement:
Write a function to generate the first n numbers in the Fibonacci sequence.
Approach:
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. We can generate the Fibonacci sequence using a simple loop.
Solution:
python
def fibonacci(n):
if n <= 0:
return []
elif n == 1:
return [0]
elif n == 2:
return [0, 1]
else:
fib = [0, 1]
for i in range(2, n):
fib.append(fib[-1] + fib[-2])
return fib
Time Complexity: O(n), where n is the number of Fibonacci numbers to generate.
Space Complexity: O(n), as we are storing the generated Fibonacci numbers in a list.
Palindrome Check
Problem Statement:
Write a function to check if a given string is a palindrome (i.e., reads the same forwards and backwards).
Approach:
To check if a string is a palindrome, we can compare the characters at the beginning and end of the string, then move inwards until we reach the middle of the string.
Solution:
python
def is_palindrome(s):
left = 0
right = len(s) – 1
while left < right:
if s[left] != s[right]:
return False
left += 1
right -= 1
return True
Time Complexity: O(n), where n is the length of the input string.
Space Complexity: O(1), as we are only using a constant amount of extra space.
Accenture Coding Questions: Intermediate-Level
Linked List Reversal
Problem Statement:
Given the head of a singly linked list, write a function to reverse the order of the nodes in the list.
Approach:
To reverse a linked list, we can use a three-pointer approach. We maintain three pointers: prev, curr, and next. We iterate through the list, updating the pointers to reverse the links between the nodes.
Solution:
python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
curr = head
while curr is not None:
next_node = curr.next
curr.next = prev
prev = curr
curr = next_node
return prev
Time Complexity: O(n), where n is the number of nodes in the linked list.
Space Complexity: O(1), as we are only using a constant amount of extra space.
Binary Search Tree Implementation
Problem Statement:
Implement a binary search tree (BST) data structure, including functions to insert, search, and delete nodes.
Approach:
A binary search tree is a tree-based data structure where each node has at most two child nodes. The left child of a node contains a value less than the node’s value, and the right child contains a value greater than the node’s value.
Solution:
python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, val):
self.root = self._insert(self.root, val)
def _insert(self, node, val):
if not node:
return TreeNode(val)
if val < node.val:
node.left = self._insert(node.left, val)
else:
node.right = self._insert(node.right, val)
return node
def search(self, val):
return self._search(self.root, val)
def _search(self, node, val):
if not node or node.val == val:
return node
if val < node.val:
return self._search(node.left, val)
else:
return self._search(node.right, val)
def delete(self, val):
self.root = self._delete(self.root, val)
def _delete(self, node, val):
if not node:
return None
if val < node.val:
node.left = self._delete(node.left, val)
elif val > node.val:
node.right = self._delete(node.right, val)
else:
if not node.left:
return node.right
elif not node.right:
return node.left
node.val = self._min_value(node.right)
node.right = self._delete(node.right, node.val)
return node
def _min_value(self, node):
while node.left:
node = node.left
return node.val
Time Complexity:
- Insert: O(log n)
- Search: O(log n)
- Delete: O(log n)
Space Complexity: O(n), where n is the number of nodes in the BST.
Breadth-First Search/Depth-First Search
Problem Statement:
Implement a function to perform Breadth-First Search (BFS) or Depth-First Search (DFS) on a graph represented as an adjacency list.
Approach:
BFS and DFS are two fundamental graph traversal algorithms. BFS explores the graph level by level, while DFS explores the graph as far as possible along each branch before backtracking.
Solution:
python
from collections import deque
def bfs(graph, start):
queue = deque([start])
visited = set([start])
while queue:
node = queue.popleft()
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
return visited
def dfs(graph, start):
stack = [start]
visited = set([start])
while stack:
node = stack.pop()
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
stack.append(neighbor)
return visited
Time Complexity:
- BFS: O(V + E), where V is the number of vertices and E is the number of edges in the graph.
- DFS: O(V + E), where V is the number of vertices and E is the number of edges in the graph.
Space Complexity:
- BFS: O(V), as we are using a queue to store the visited nodes.
- DFS: O(V), as we are using a stack to store the visited nodes.
Accenture Coding Questions: Advanced-Level
Dynamic Programming Problem (e.g., Knapsack Problem)
Problem Statement:
Given a set of items, each with a weight and a value, and a maximum weight capacity, determine the maximum total value of items that can be included in a knapsack without exceeding the weight limit.
Approach:
The Knapsack problem is a classic dynamic programming problem. We can solve it using a 2D array to store the maximum value that can be obtained for each weight capacity and number of items.
Solution:
python
def knapsack(weights, values, capacity):
n = len(weights)
dp = [[0] * (capacity + 1) for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i – 1] <= w:
dp[i][w] = max(dp[i – 1][w], dp[i – 1][w – weights[i – 1]] + values[i – 1])
else:
dp[i][w] = dp[i – 1][w]
return dp[n][capacity]
Time Complexity: O(n * capacity), where n is the number of items and capacity is the maximum weight capacity.
Space Complexity: O(n * capacity), as we are using a 2D array to store the intermediate results.
Graph Algorithm (e.g., Shortest Path)
Problem Statement:
Given a weighted graph, find the shortest path between two specified nodes.
Approach:
One of the most popular algorithms for finding the shortest path in a weighted graph is Dijkstra’s algorithm. It uses a greedy approach to find the shortest path by repeatedly selecting the node with the smallest distance from the unvisited nodes.
Solution:
python
import heapq
def dijkstra(graph, start, end):
distances = {node: float(‘inf’) for node in graph}
distances[start] = 0
heap = [(0, start)]
while heap:
current_distance, current_node = heapq.heappop(heap)
if current_distance > distances[current_node]:
continue
if current_node == end:
return current_distance
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(heap, (distance, neighbor))
return -1
Time Complexity: O((V + E) log V), where V is the number of vertices and E is the number of edges in the graph.
Space Complexity: O(V), as we are using a dictionary to store the distances and a heap to keep track of the nodes to visit.
Remember, these are just a few examples of the types of coding questions you may encounter in the Accenture coding round. It’s essential to practice a wide range of questions, from basic data structures and algorithms to more advanced topics, to improve your problem-solving skills and increase your chances of success in the Accenture coding interview.
Additional Tips for Accenture Coding Interview Success
Getting ready for a coding interview at Accenture takes more than knowing how to code. Here are some more tips to help you do well and get the job.
1) Time Management:
- Set a Timer: When you practice coding problems, use a timer. This helps you get used to the pressure of a real interview.
- Learn to Prioritise: You won’t always have time to solve everything perfectly. Know which parts of a problem are most important to solve first.
- Don’t Panic: If you get stuck, don’t waste too much time on one thing. Move on and come back to it later if you have time.
2) Testing Your Code:
- Think of Test Cases: Before you start writing code, think of different inputs you can test your code with.
- Check for Edge Cases: Don’t just test the obvious cases. Think of unusual inputs that could break your code.
- Test as You Go: Don’t wait until the end to test everything. Test small parts of your code as you write them.
3) Communication:
- Talk Through Your Steps: Explain what you’re doing as you solve the problem. This shows the interviewer how you think.
- Ask Questions: If you don’t understand something, ask for clarification. It’s better to ask than to guess wrong.
- Be Honest: If you don’t know the answer, say so. But show that you’re willing to learn and try to solve the problem.
4) Online Resources:
- iScalePro: This website has lots of practice problems that are similar to what you might see in an Accenture interview.
- LeetCode: This website is popular with many software engineers. It has a large collection of coding problems and a community to help you.
- HackerRank: This website hosts coding challenges and competitions. It’s a good way to practise and see how you compare to others.
Conclusion
You’ve just gotten a glimpse into the types of coding questions Accenture may ask. Preparing for these interviews takes time and practice. To further hone your coding skills and boost your confidence, try iScalePro.
This platform offers a wide range of coding challenges similar to those you might encounter in your Accenture interview. With iScalePro, you can practise, learn, and prepare yourself to ace your Accenture coding interview.
Accenture Coding Interview FAQs
1) Does Accenture repeat coding questions?
Yes, Accenture does repeat coding questions. The company has a pool of coding questions that they use for interviews. However, the specific questions you will be asked will depend on your role and the team you are interviewing with.
2) Is Accenture coding round hard?
The difficulty of the Accenture coding round varies. Some candidates find it easy, while others find it challenging. The difficulty of the round also depends on your experience and skills. However, if you are well-prepared and have a strong understanding of data structures and algorithms, you should be able to do well.
3) How to clear Accenture coding assessment?
Here are some tips on how to clear the Accenture coding assessment:
- Practice coding regularly. The more you practice, the better you will become at coding.
- Learn data structures and algorithms. Data structures and algorithms are essential for coding interviews. Make sure you have a strong understanding of these concepts.
- Solve coding problems. Practice solving coding problems on online platforms like LeetCode and HackerRank.
- Be prepared to explain your code. You will need to be able to explain your code to the interviewer. Make sure you can articulate your thought process clearly.
4) Does Accenture have coding?
Yes, Accenture has coding. Coding is an important part of many roles at Accenture. If you are applying for a role that requires coding skills, you will likely be asked to complete a coding assessment as part of the interview process.