Coding questions are a big part of the TCS Digital interview. You need to answer them well to get the job. But finding the right practice questions can be tough. There’s a lot of information out there, and it’s not always clear which questions are most relevant.
This article can help. We’ve put together a list of common TCS Digital coding questions, along with clear answers and explanations. So you can practise and get ready to impress at your interview.
TCS Digital Coding Question Categories
TCS Digital assessments often include a coding section to evaluate your programming skills. Here’s a breakdown of common question categories you might encounter:
1) String Manipulation:
These questions involve working with text data. You’ll need to identify characters, substrings, or manipulate strings entirely. Examples include:
- Counting unique characters: Write code to find the number of unique characters present in a given string.
- Reversing a string: Develop code that reverses the order of characters in a string.
- Checking for palindrome: Create a program that determines if a string reads the same backward as forward (e.g., “racecar”).
2) Number Theory:
This category focuses on numerical operations, divisibility rules, and prime numbers. Be prepared for questions like:
- Finding prime factors: Write code to identify the prime factors of a given number.
- Calculating GCD/LCM: Develop a program to find the Greatest Common Divisor (GCD) or Least Common Multiple (LCM) of two numbers.
- Checking for Armstrong number: Create code that determines if a number is an Armstrong number (a number whose sum of cubed digits is equal to the original number, e.g., 153).
3) Arrays and Lists:
These questions test your ability to work with data structures like arrays and lists. You might encounter problems involving:
- Finding the maximum/minimum element: Write code to identify the largest or smallest element within an array or list.
- Reversing an array: Develop a program that reverses the order of elements in an array.
- Removing duplicates: Create code that removes duplicate elements from an array or list.
4) Problem-Solving:
This category goes beyond basic coding syntax and assesses your logical reasoning and problem-solving skills using code. Examples include:
- Finding the missing number in a sequence: Write code to identify the missing number in a given sequence that follows a specific pattern.
- Finding all pairs with a given sum: Develop a program to find all pairs of numbers within an array that add up to a specific value.
- Pattern printing: Create code that prints specific patterns using characters or numbers (e.g., a pyramid, a triangle).
Move on to the next section to see real TCS Digital coding questions with solutions!
TCS Digital Coding Questions & Answers
Ready to dive into practice? Here are 15 TCS Digital coding questions along with clear explanations and solutions. These will help you test your skills and understand how to approach different coding challenges.
1) Rotate Array
Problem: Given an array Arr[ ] of N integers and a positive integer K. The task is to cyclically rotate the array clockwise by K.
Note: Keep the first element of the array unaltered.
Example:
N = 5
Arr[] = {10, 20, 30, 40, 50}
K = 2
Output: 40 50 10 20 30
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
int main() {
int n, k;
cin >> n >> k;
vector<int> arr(n);
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
for (int i = 1; i <= k; i++) {
int temp = arr[n-1];
for (int j = n-1; j > 0; j–) {
arr[j] = arr[j-1];
}
arr[0] = temp;
}
for (int i = 0; i < n; i++) {
cout << arr[i] << ” “;
}
cout << endl;
return 0;
}
2) Prisoner’s Dilemma
Problem: There are n prisoners standing in a circle. The jailer starts passing out treats from the prisoner standing at position s. He passes one treat to each prisoner, one by one, moving clockwise around the circle. After a prisoner receives a treat, they leave the line. The jailer keeps passing out treats until all prisoners have been executed.
Given n, m, and s, determine the number of prisoners that receive a treat.
Input Format:
The first line contains an integer t, denoting the number of test cases.
The next t lines each contain 3 space-separated integers:
n: the number of prisoners
m: the number of sweets
s: the chair number to start passing out treats at
Output Format:
For each test case, print the number of prisoners that receive a treat.
Example:
n = 5, m = 2, s = 1
Output: 3
Solution:
python
define prisonersDilemma(n, m, s):
prisoners = 1
while True:
s = (s + m – 1) % n
if s == 0:
return prisoners
prisoners += 1
3) Kadane’s Algorithm
Problem: Given an array Arr[ ] of N integers. Find the contiguous sub-array(containing at least one number) which has the maximum sum and return its maximum sum.
Example:
N = 5
Arr[] = {1,2,-1,-2,1}
Output: 3
Explanation: The sub-array [1,2] has the maximum sum 3.
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
int maxSubarraySum(int arr[], int n) {
int max_so_far = INT_MIN, max_ending_here = 0;
for (int i = 0; i < n; i++) {
max_ending_here = max_ending_here + arr[i];
if (max_so_far < max_ending_here)
max_so_far = max_ending_here;
if (max_ending_here < 0)
max_ending_here = 0;
}
return max_so_far;
}
int main() {
int n;
cin >> n;
int arr[n];
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
cout << maxSubarraySum(arr, n) << endl;
return 0;
}
4) Reverse Words in a String
Problem: Given a String S, reverse the order of words in it. Words are separated by dots.
Example:
S = “i.like.this.program.very.much”
Output: “much.very.program.this.like.i”
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
string reverseWords(string s) {
vector<string> words;
string word = “”;
for (int i = 0; i < s.length(); i++) {
if (s[i] == ‘.’) {
words.push_back(word);
word = “”;
} else {
word += s[i];
}
}
words.push_back(word);
string result = “”;
for (int i = words.size()-1; i >= 0; i–) {
result += words[i];
if (i != 0) {
result += “.”;
}
}
return result;
}
int main() {
string s;
getline(cin, s);
cout << reverseWords(s) << endl;
return 0;
}
5) Merge Two Sorted Linked Lists
Problem: Given two sorted linked lists consisting of N and M nodes respectively. The task is to merge both of the lists (in-place) and return the head of the merged list.
Example:
N = 4, M = 3
List1 = 1->2->3->4
List2 = 2->3->20
Output: 1->2->2->3->3->4->20
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* next;
Node(int x) {
data = x;
next = NULL;
}
};
Node* mergeTwoLists(Node* l1, Node* l2) {
if (l1 == NULL) return l2;
if (l2 == NULL) return l1;
if (l1->data < l2->data) {
l1->next = mergeTwoLists(l1->next, l2);
return l1;
} else {
l2->next = mergeTwoLists(l1, l2->next);
return l2;
}
}
int main() {
// Create linked lists
Node* l1 = new Node(1);
l1->next = new Node(2);
l1->next->next = new Node(3);
l1->next->next->next = new Node(4);
Node* l2 = new Node(2);
l2->next = new Node(3);
l2->next->next = new Node(20);
Node* merged = mergeTwoLists(l1, l2);
// Print merged list
while (merged != NULL) {
cout << merged->data << ” “;
merged = merged->next;
}
cout << endl;
return 0;
}
6) Reverse a Linked List
Problem: Given a linked list of N nodes. The task is to reverse this list.
Example:
N = 4
Linked List: 1->2->3->4
Output: 4->3->2->1
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* next;
Node(int x) {
data = x;
next = NULL;
}
};
Node* reverseList(Node* head) {
Node* prev = NULL;
Node* curr = head;
while (curr != NULL) {
Node* next_node = curr->next;
curr->next = prev;
prev = curr;
curr = next_node;
}
return prev;
}
int main() {
// Create linked list
Node* head = new Node(1);
head->next = new Node(2);
head->next->next = new Node(3);
head->next->next->next = new Node(4);
Node* reversed = reverseList(head);
// Print reversed list
while (reversed != NULL) {
cout << reversed->data << ” “;
reversed = reversed->next;
}
cout << endl;
return 0;
}
7) Detect Loop in Linked List
Problem: Given a linked list, check if the linked list has a loop. A linked list has a loop if any node in the linked list points to a previous node in the linked list. The function returns true if a loop is present. Otherwise, it returns false.
Example:
Linked List: 1->2->3->4->5->2 (The last node points back to the second node making it a loop)
Output: true
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* next;
Node(int x) {
data = x;
next = NULL;
}
};
bool detectLoop(Node* head) {
if (head == NULL || head->next == NULL) {
return false;
}
Node* slow = head;
Node* fast = head->next;
while (slow != fast) {
if (fast == NULL || fast->next == NULL) {
return false;
}
slow = slow->next;
fast = fast->next->next;
}
return true;
}
int main() {
// Create linked list with loop
Node* head = new Node(1);
head->next = new Node(2);
head->next->next = new Node(3);
head->next->next->next = new Node(4);
head->next->next->next->next = head->next;
if (detectLoop(head)) {
cout << “Loop detected” << endl;
} else {
cout << “No loop detected” << endl;
}
return 0;
}
8) Reverse a String
Problem: Given a string S, reverse the string without affecting special characters.
Example:
S = “a!!!b.c.d,e’f,ghi jkl!mn pqr s,t$u v wxy z”
Output: “a!!!b.c.d,e’f,ihg jkl!nm rqp s,t$u v wxy z”
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
string reverseString(string s) {
int left = 0, right = s.length() – 1;
while (left < right) {
if (!isalpha(s[left])) {
left++;
} else if (!isalpha(s[right])) {
right–;
} else {
swap(s[left], s[right]);
left++;
right–;
}
}
return s;
}
int main() {
string s = “a!!!b.c.d,e’f,ghi jkl!mn pqr s,t$u v wxy z”;
cout << reverseString(s) << endl;
return 0;
}
9) Reverse a Stack
Problem: Given a stack, the task is to reverse the stack without using any extra space.
Example:
Stack: 1->2->3->4->5
Output: 5->4->3->2->1
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
void reverseStack(stack<int>& st) {
if (st.empty()) {
return;
}
int top_element = st.top();
st.pop();
reverseStack(st);
stack<int> temp;
while (!st.empty()) {
temp.push(st.top());
st.pop();
}
st.push(top_element);
while (!temp.empty()) {
st.push(temp.top());
temp.pop();
}
}
int main() {
stack<int> st;
st.push(1);
st.push(2);
st.push(3);
st.push(4);
st.push(5);
reverseStack(st);
while (!st.empty()) {
cout << st.top() << ” “;
st.pop();
}
cout << endl;
return 0;
}
10) Reverse a Queue
Problem: Given a queue, the task is to reverse the queue without using any extra space.
Example:
Queue: 1->2->3->4->5
Output: 5->4->3->2->1
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
queue<int> reverseQueue(queue<int>& q) {
if (q.empty()) {
return q;
}
int front_element = q.front();
q.pop();
q = reverseQueue(q);
q.push(front_element);
return q;
}
int main() {
queue<int> q;
q.push(1);
q.push(2);
q.push(3);
q.push(4);
q.push(5);
q = reverseQueue(q);
while (!q.empty()) {
cout << q.front() << ” “;
q.pop();
}
cout << endl;
return 0;
}
11) Merge K Sorted Linked Lists
Problem: Given K sorted linked lists of different sizes. The task is to merge them in such a way that after merging they will be a single sorted linked list.
Example:
K = 3
Linked List 1: 1->2->3
Linked List 2: 4->5->6
Linked List 3: 7->8->9
Output: 1->2->3->4->5->6->7->8->9
Solution:
cpp
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* next;
Node(int x) {
data = x;
next = NULL;
}
};
Node* mergeKLists(Node* arr[], int k) {
if (k == 0) {
return NULL;
}
priority_queue<pair<int, Node*>, vector<pair<int, Node*>>, greater<pair<int, Node*>>> pq;
for (int i = 0; i < k; i++) {
if (arr[i] != NULL) {
pq.push({arr[i]->data, arr[i]});
}
}
Node* dummy = new Node(0);
Node* tail = dummy;
while (!pq.empty()) {
Node* curr = pq.top().second;
pq.pop();
tail->next = curr;
tail = tail->next;
if (curr->next != NULL) {
pq.push({curr->next->data, curr->next});
}
}
return dummy->next;
}
int main() {
int k = 3;
Node* arr[k];
// Create linked lists
arr[0] = new Node(1);
arr[0]->next = new Node(2);
arr[0]->next->next = new Node(3)
arr[1] = new Node(4);
arr[1]->next = new Node(5);
arr[1]->next->next = new Node(6);
arr[2] = new Node(7);
arr[2]->next = new Node(8);
arr[2]->next->next = new Node(9);
Node* head = mergeKLists(arr, k);
// Print merged list
while (head != NULL) {
cout << head->data << ” “;
head = head->next;
}
cout << endl;
return 0;
}
12) Longest Common Prefix
Problem: Write a function to find the longest common prefix string amongst an array of strings.
Example:
Input: [“flower”, “flow”, “flight”]
Output: “fl”
Solution:
python
def longestCommonPrefix(strs):
if not strs:
return “”
prefix = strs[0]
for s in strs[1:]:
while not s.startswith(prefix):
prefix = prefix[:-1]
if not prefix:
return “”
return prefix
# Example usage
print(longestCommonPrefix([“flower”, “flow”, “flight”])) # Output: “fl”
13) Valid Parentheses
Problem: Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid.
Example:
Input: “()[]{}”
Output: true
Solution:
python
def isValid(s):
stack = []
mapping = {“)”: “(“, “}”: “{“, “]”: “[“}
for char in s:
if char in mapping:
top_element = stack.pop() if stack else ‘#’
if mapping[char] != top_element:
return False
else:
stack.append(char)
return not stack
# Example usage
print(isValid(“()[]{}”)) # Output: True
14) Two Sum
Problem: Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target.
Example:
Input: nums = [2, 7, 11, 15], target = 9
Output: [0, 1]
Solution:
python
def twoSum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target – num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
# Example usage
print(twoSum([2, 7, 11, 15], 9)) # Output: [0, 1]
15) Find the Missing Number
Problem: Given an array containing n distinct numbers taken from 0, 1, 2, …, n, find the one number that is missing from the array.
Example:
Input: [3, 0, 1]
Output: 2
Solution:
python
def missingNumber(nums):
n = len(nums)
return n * (n + 1) // 2 – sum(nums)
# Example usage
print(missingNumber([3, 0, 1])) # Output: 2
These problems cover a range of algorithms and data structures, including array manipulation, string processing, stack and queue operations, and linked list handling. Each solution is provided with example usage to illustrate how to implement and test the function.
Ready to boost your preparation? Check out the next section for helpful tips!
Tips for Preparing for TCS Digital Coding Questions
Landing a job at TCS Digital requires strong coding skills. The coding assessment is a crucial step in the selection process. This guide provides valuable tips to help you excel in this assessment.
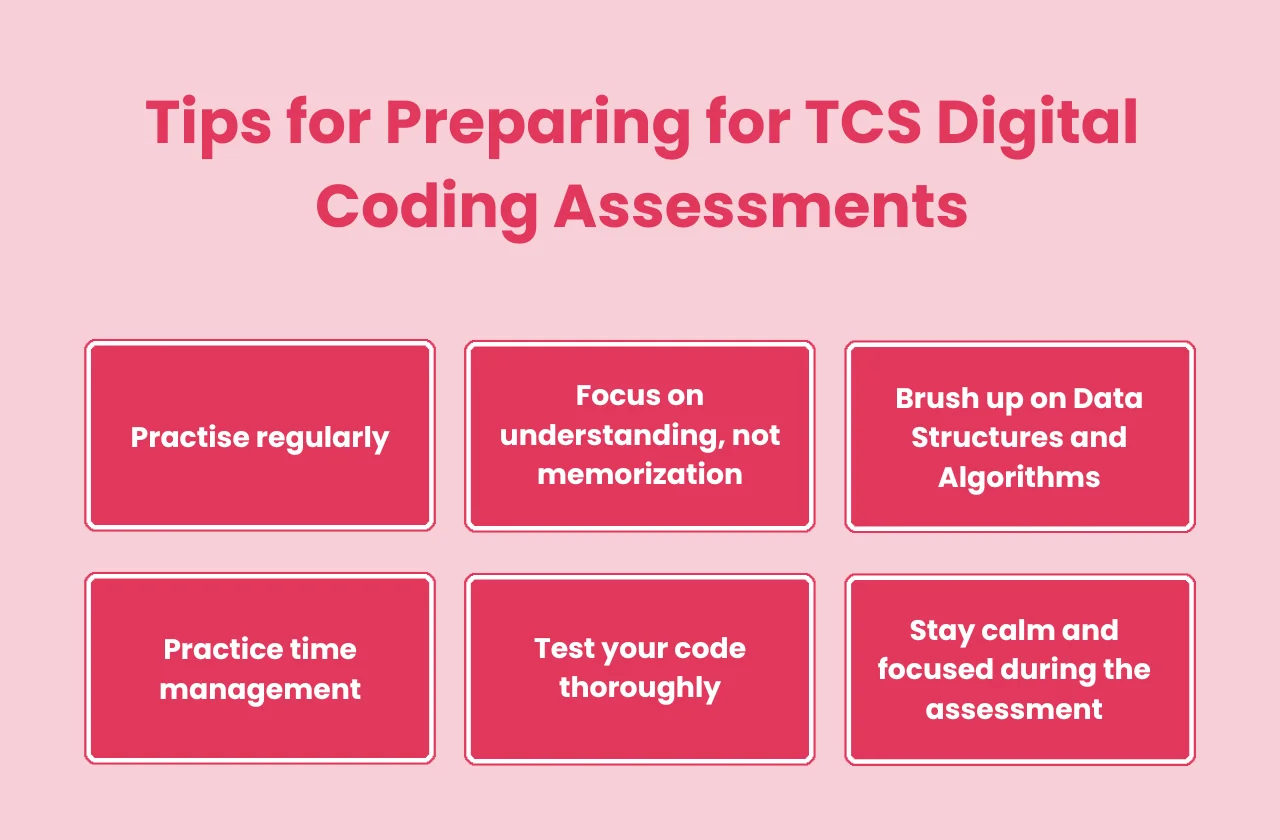
1) Practise regularly:
Regular practice is essential for success. Online platforms like iScalePro offer a vast collection of coding problems similar to those encountered in the TCS Digital assessment. These platforms provide solutions and explanations, allowing you to learn from different approaches.
2) Focus on understanding, not memorization:
While memorising code snippets might seem tempting, it’s far more beneficial to understand the logic behind them. Grasping the core concepts of algorithms used in solutions will equip you to tackle new problems effectively.
3) Brush up on Data Structures and Algorithms:
Data structures and algorithms are the building blocks of efficient coding. Understanding how different data structures work (like arrays, linked lists, trees) and common algorithms (sorting, searching) will empower you to solve problems efficiently. Many online resources and tutorials are available to strengthen your foundation in these areas.
4) Practice time management:
The TCS Digital coding assessment is time-bound. To perform well under pressure, practice solving problems under timed conditions. Set a timer when working on practice problems online. This will help you develop the ability to analyse, code, and test your solutions effectively within the given timeframe.
5) Test your code thoroughly:
Don’t assume your code works perfectly after writing it. Always test your code with various inputs, including edge cases (unusual or unexpected inputs). Platforms like iScalePro often provide multiple test cases, allowing you to verify if your code produces the correct output for different scenarios. Thorough testing ensures your code is reliable and handles diverse situations.
6) Stay calm and focused during the assessment:
Feeling nervous during the assessment is natural. However, staying calm is crucial for clear thinking. Take deep breaths if you feel overwhelmed. Approach each question strategically. Read the problem statement carefully, identify the core problem, and then choose the most suitable data structures and algorithms to solve it.
By following these tips and dedicating yourself to regular practice, you can significantly increase your chances of success in the TCS Digital coding assessment.
Conclusion
Acing the coding portion of your TCS Digital interview is important. This article provided you with practice questions like finding unique digits or counting prime pairs, along with solutions.
Remember, the more you practise, the more confident you’ll feel. Take your prep to the next level with iScalePro, our practice tool designed to simulate real interview questions. iScalePro will help you improve your coding skills and land your dream job at TCS Digital.
TCS Digital Coding FAQs
1) Are TCS coding questions easy?
TCS coding questions are not easy. They require strong problem-solving skills and knowledge of programming concepts. However, they are not impossible to solve. With practice and a good understanding of algorithms and data structures, you can crack them.
2) Is TCS digital tough to crack?
TCS digital is tough to crack. It is a competitive exam that requires thorough preparation. The coding questions are challenging, and the time limit is tight. However, with the right strategy and hard work, you can crack it.
3) Is TCS digital exam easy?
No, the TCS digital exam is not easy. It is a competitive exam that requires a high level of preparation. The coding questions are challenging, and the time limit is tight.
4) What are the questions asked in TCS digital?
TCS digital coding questions are typically based on algorithms and data structures. Some common topics include:
- Arrays
- Linked lists
- Stacks
- Queues
- Trees
- Graphs
- Sorting
- Searching
- Dynamic programming
- Greedy algorithms
The questions can be in the form of coding problems or puzzles. They may require you to write complete code or just the logic.